Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial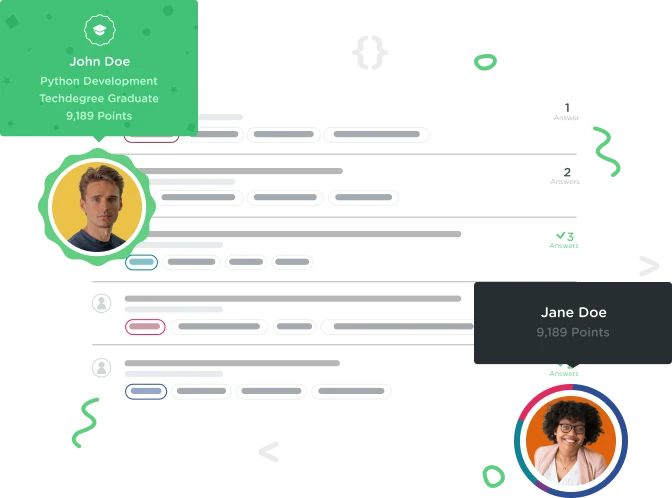
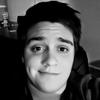
Noah J Neal
1,850 PointsRandom number generator (alternate code?)
I used this method instead of the given answer, which worked.
var low = prompt('Enter a number'); var high = prompt('Enter another larger number');
alert(Math.floor(Math.random() * parseInt(high)) + parseInt(low));
Is this an acceptable method?
2 Answers
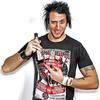
Andreas Nyström
8,887 PointsHi.
No this is not correct. What your math actually does is this:
Math.floor() = take a decimal value and drop it down to the lowest integer (3.9 becomes 3, 4.5 becomes 4 and so on). Math.random() = generates a number from 0 up to but no including 1 (such as 0.44433 and 0.999) You ask the prompts right, but the equation isnt right.
Because (Math.random() * the high number) + low number is not a random number. For example:
Math.random() = 0.4 High number = 10 Low number = 3
This will end up like this:
(0.4 * 10) + 3 = 7
So your low number doesnt really do anything except adding to the random number from the high number. What it should look like is this:
var low = prompt('Enter a number');
var high = prompt('Enter another larger number');
alert(Math.floor(Math.random() * (parseInt(high) - parseInt(low) +1) + parseInt(low));
This is very hard to read because you're not using variables. Instead you're using the parseInt in the alert instead of doing it before. But that's up to you. I would try to use variables as much as possible instead to get the understanding of it. If you don't understand the math of this, I can try to explain it, just hit me up in this thread.
For example:
var input1 = prompt('Enter a number');
var input2 = prompt('Enter another larger number');
var lowNumber = parseInt(input1);
var highNumber = parseInt(input2);
var randomNumber = Math.floor(Math.random() * (highNumber - lowNumber +1) + lowNumber
alert(randonNumber);
Isnt this easier to read? And also now you can do other stuff with your input, your randomNumber and so on :).

Sharath Chandra
3,957 PointsAndreas Nyström Could you please explain the math in the equation.
var randomNumber = Math.floor(Math.random() * (highNumber - lowNumber +1) + lowNumber

acca acgac
Front End Web Development Techdegree Student 7,208 PointsHi Sharath,
The post is lengthy but I think it worths your attention. The reason it's a little hard to understand is that all the work is done by one line of code. It would be better if the code from the instructor was broken down into more variables to mark each stage of solving. It takes me a few days to understand this and generalize the concept before explaining this to you, so please be patient.
I would try to explain this to you by first talking about the first assignment, which is to generate a random number from 1 to the number input by the user.
Say, my input number is 8: var inputNumber = 8; var randomNumber = Math.random(); var multipliedNumber = randomNumber * inputNumber; // now the range should be [0; 8)
Math.random() generates a random number from 0 (inclusive) up to but not include 1, or by math notation of [0; 1). If I multiply the inputNumber by randomNumber (its range is [0; 1)), I would get the minimum random number of 0 and the maximum random number of 7.9999999999999999. That's why we don't get 8. If I "floor" this by using Math.floor(), I would get the minimum integer of 0 and the maximum integer of 7. Since we want the random number from 1 to 8. We'll add 1 to the result to get the final random number between the range [1;8]. The problem is now solved.
Now we move on to the second assignment. The idea behind its solution is to generate a random number between 0 and the difference between highNumber and lowNumber [highNum - lowNum]. Next, add this random number to the lowNumber.
Take a look at my example below.
lowNumber = 16 highNum = 20 randomNum = Math.random() theDifference = 4 (result of 20 - 16) desiredRandomNumber = [0; 4] finalRandomNumber = minNum + desireRandomNumber //
If desiredRandomNumber is 0, 1, 2, 3, or 4, we'll get the finalRandomNumber of 16, 17, 18, 19, or 20 respectively. I hope you're with me at this point.
The only difference between these 2 assignments, except the last step of adding 1 to lowNumber, is that we want number 0 to be included, not like the first assignment where we need 1. Note that if you want 0 to be included, the code is different. That's why many people were confused when moving to the second part, including me a couple days ago. You'll see what I mean and how the code would need to be changed if you want to generate a random number from 0 to 8. Look at Andreas code and try to understand why we need to plus 1 when subtracting lowNumber from highNumber. The addition of 1 is where the magic happens.
Now, look at the fictional variables I wrote above. If I generate a random number stored in randomNum by Math.random() and multiply it by theDifference, what is the range I would get? I would be [0; 3.9999999999999999]. If I "floor" this result, I would get [0; 3], which is not [0; 4] as wished. That's why we need to add 1 BEFORE we "floor" it. If I add one to theDifference (20 - 16 + 1 or (highNumber - lowNum + 1)), then multiply theDifference by randomNum, I'll get the desiredRandomNumber in the range of [0; 4.9999999999999999]. From this point, "flooring" it will result in the range of [0; 4].
So we "floor" the whole thing:
Math.floor( Math.random() * (highNumber - lowNumber + 1 )) // Andreas missed the additional ")"
After getting the desiredRandomNum from the desired range, we add it to the lowNumber to get the finalRandomNumber.
I hope this makes sense to you.
P/S: If you want to grab a better understanding and make it more difficult, try generating a random number from the range of [0; 21] and [1; 21] or any number you like by using both Math.floor() and Math.ceil() (4 possible solutions: 2 for 0 up and 2 for 1 up). Then go ahead and generalize the problem by receiving the input from the user. Then finally, come back to this assignment. You'll see it's easier. That process took me 2 days and I think I got smarter by doing that. You might wanna give it a try.