Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial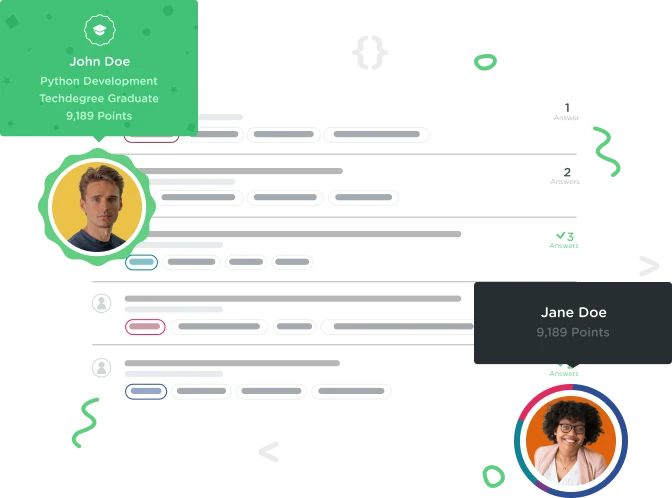

H Yang
2,066 PointsRe: Printing
Instead of building a print function the way the instructor did here:
function print(message) {
var outputDIV = document.getElementById("output");
outputDIV.innerHTML = message;
}
function buildList(arr) {
var listHTML = "<ol>";
for (var i= 0; i < arr.length; i += 1) {
listHTML += "<li>" + arr[i] + "</li>";
}
listHTML += "</ol>";
return listHTML;
}
html = "You got " + correctAnswers + " question(s) right."
html += "You got these questions correct:";
html += buildList(correct);
html += "You got these questions wrong:";
html += buildList(wrong);
print(html);
I built mine this way:
function print(message) {
var outputDIV = document.getElementById("output" );
outputDIV.innerHTML = message;
}
function printList (list) {
var listHTML = "<ol>";
for ( var i=0; i < list.length; i += 1) {
listHTML += "<li>" + list[i] + "</li>";
}
listHTML += "</ol>";
print(listHTML);
}
html = "You got " + correctAnswers + " question(s) right.";
print(html);
print("You got these questions correct:");
printList(correctQuestions);
print("You got these questions incorrect:");
printList(incorrectQuestions);
Note that instead of using return at the end of the buildList function, i used the print at the end of mine. Also at the end of my print function, instead of concatenating all the statements together into the html statement and printing that one statement, I tried to print all of mine separately.
My code didn't work. What am I doing wrong? For reference, here is my whole code
var questions = [
['How many states are in the United States?', 50],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
var correctAnswers = 0;
var correctQuestions = [];
var incorrectQuestions = [];
var question;
var answer;
var response;
var html;
function print(message) {
var outputDIV = document.getElementById("output");
outputDIV.innerHTML = message;
}
function printList (list) {
var listHTML = "<ol>";
for ( var i=0; i < list.length; i += 1) {
listHTML += "<li>" + list[i] + "</li>";
}
listHTML += "</ol>";
print(listHTML);
}
for (var i = 0; i < questions.length; i += 1) {
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
response = parseInt(response);
if (response === answer) {
correctQuestions.push(question);
correctAnswers += 1;
} else {
incorrectQuestions.push(question);
}
}
html = "You got " + correctAnswers + " question(s) right.";
print(html);
print("You got these questions correct:");
printList(correctQuestions);
print("You got these questions incorrect:");
printList(incorrectQuestions);
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Henry,
Having the buildList function return the list html makes the code more flexible.
You can still print the return value right away if that's what you need to do but you can also do further processing on that value and print at a later time.
For your issue with separate prints, the print function is replacing the contents of the div with the new message passed in.
This is why all the html is built up and then written at once to the div.
H Yang
2,066 PointsH Yang
2,066 PointsThank you for the help Jason