Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial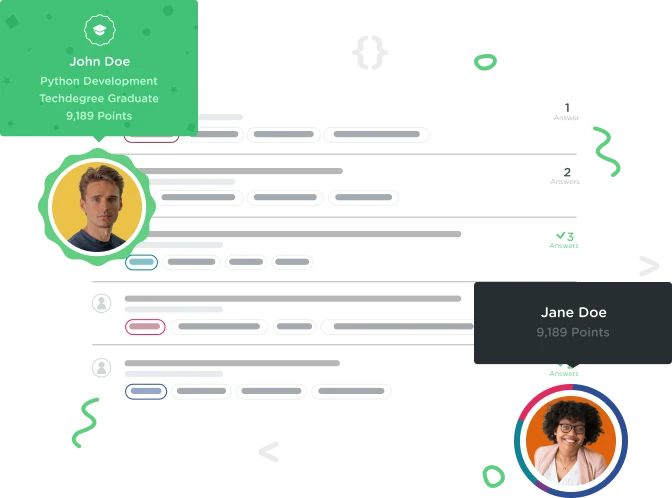
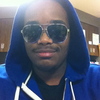
Roger Green
643 PointsReally Confused
I am truly confused with the video I just watched. I don't understand some of the syntax they're using such as void,NSLog, NSArray, etc and Im confused about the order they put them in.
2 Answers
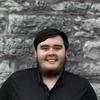
Michael Hulet
47,913 PointsI'll try to explain them all..
void
is the return type for a function. It indicates that the function does not, in fact, return anything. For example, this method will simply print the phrase "Hello World!" to the console, but the method itself will not return anything:
+(void)helloWorld{
NSLog(@"Hello World!");
//Notice that there's no return statement; just the call to NSLog
}
This seems like a good time to introduce NSLog()
. You can think of it as C's printf()
, but for Objective-C, because it works exactly the same way and does exactly the same thing: print a string of text to the console. The difference between the 2 is that NSLog()
accepts an NSString
(denoted by the @
right before the first quotation mark), instead of a C char
array. Since C doesn't actually have a data type for a string (only a single character), Apple made one for Objective-C, and it's called NSString. You'll learn all about those later on. The other thing unique to NSLog()
is that you can use the %@
placeholder. What this means is that you can pass in an object as an argument (after the first, required NSString
), and then the description
method will be called on the object you passed in, and the return of that method will be pasted in where the %@
is. It's exactly the same as %d
for C double
s or int
s, but %@
is by far the most commonly used placeholder in Objective-C. To demonstrate this, the following code is just another way to do the exact same thing demonstrated above, in the last block of code I showed you:
+(void)helloWorld{
NSString *text = @"Hello World!";
NSLog(@"%@", text);
}
Finally, NSArray
is exactly the same thing as a C array, but for Objective-C. The difference is that a C array can hold a specific data type (defined when you're declaring the array), but NSArray
only holds objects. This might sound limiting, but considering that objects are by far the most common type of variable you're going to be working with, it's actually very helpful. To demonstrate, the following code should just print a short grocery list to the console:
+(void)groceries{
//Notice how the NSArray is assigned using "@" syntax. Also, this array only holds NSStrings (which are objects), but you could hold any object type you like in an NSArray, and even intermingle them
NSArray *groceries = @[@"bread",@"milk",@"eggs"];
NSLog(@"%@", groceries);
}
I hope this helps, but reply to this if you have any questions!

Chris Shaw
26,676 PointsHi Roger,
There is no specific order NSLog
and NSArray
need to go in but void
is slightly different as it denotes that no value for example will be returned from a method.
I would recommend you keep watching the videos over and over along with reading Apple's documentation which is really helpful.