Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial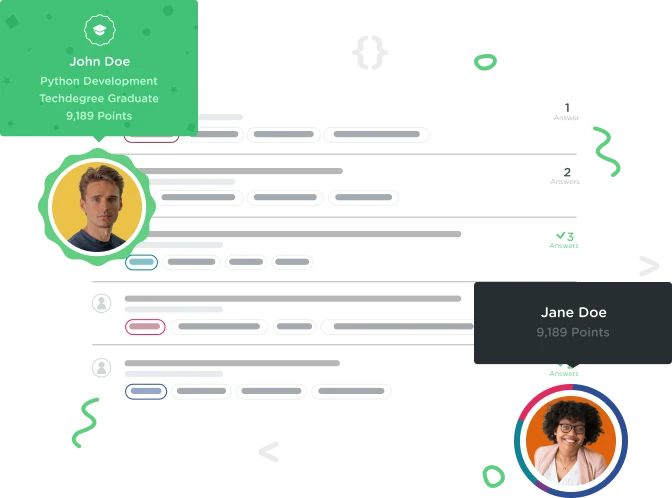

Jan M
1,053 PointsReally need help understanding this, could someone explain in detail?
I started this course a few weeks back and have been doing my (almost) daily dose of Python since then . However I seemed to have gotten lost somewhere on the track and now can't solve any of the coding questions without having to Google for the answers. I do understand the general stuff like lists and what a dictionary is, however I still cant really wrap my head around loops and the similiar. Could someone please take the time and explain how loops (for example as used in this question) work and how/when I use them. I would love to be able to use Python myself and be able to automate tasks, however right now I am just really frustrated about my almost non existent progression.
Thanks a lot in advance :) :) :)
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(dicts):
most_class = "" # holds the name of teach with most class
max_count = 0 # max counter for classes
for teacher in dicts:
if len(dicts[teacher]) > max_count:
max_count = len(dicts[teacher])
most_class = teacher
return most_class
def num_teachers(teachers):
busy_teacher = 0
for key in teachers.keys():
busy_teacher += 1
return busy_teacher
def stats(dict):
newList = []
for k,v in dict.items():
newString = [k, int(len(v))]
newList.append(newString)
return newList
3 Answers
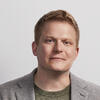
Keith Whatling
17,759 PointsIts fine, it takes a while but its kinda like arrays in VBA if you have ever done any of that. Loops, dicts and lists are really super powerful so no stupid questions here, the better you understand them the more power you'll have.
I have written and made a living from loops and arrays with VBA in the past, they can massively simplify and reduce the time to do repetitive tasks.
Ok so python does not know what yo mean by key, it is just a really good naming convention :)
Lets break down the first one,
dictionary is referring to the entire dictionary. Put one of the keys in the dictionary in the [] will return the values of the key inside that part of the dictionary.
To be really clear, lets really think of a real book based dictionary.
So I want to look up the meaning of some words in my oxford dictionary. I want to look up ice cream and sausage.
In my dictionary I could loop through all the words looking for the key (the words i'm looking for ice cream and sausage) or could just say dictionary['sausage'].
This would 'return'=
"minced pork, beef, or other meats, often combined, together with various added ingredients and seasonings, usually stuffed into a prepared intestine or other casing and often made in links. "
The best way to understand them is to make a dict and a list and start playing with them. make a dict. start simple with key value like a quick phonebook = {'mum': 079561234567, 'dad': 077937654432, 'wife': 019201234567}
so copy the code into python and comment out sections to see what happens.
phonebook = {'mum': '079561234567', 'dad': '077937654432', 'wife': '019201234567'}
#print the dictionary to check what comes out
print(phonebook)
#now I just want my mums number i should expect to see 079561234567
print(phonebook['mum'])
#now lets see whos number I have in my phonebook, I expect an output of, mum, dad, wife.
for familey_member in phonebook:
print(familey_member)
# now lets just print out the numbers three different ways.
#Basic
print(phonebook['mum'])
print(phonebook['dad'])
print(phonebook['wife'])
#with a loop with the familey_member(the key) in the [] after dictionary.
for familey_member in phonebook:
print(phonebook[familey_member])
#with a loop with items()
for familey_member, phone_number in phonebook.items():
print(phone_number)
#now lets print out everything that looks nice.
for familey_member, phone_number in phonebook.items():
print(familey_member, phone_number)
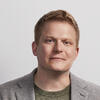
Keith Whatling
17,759 PointsOk
Dicts are great fun! However starting them can be a little tricky, I came a cropper with the same question.
The bit you are missing is to use dict.items().
So this gives you back the key AND the values. So to explain further
If I have a dict like this {'keith': ['ginger', 'English', 'Chubby'], 'mywife': ['blonde', 'polish', 'slim']}
So we have a dict that has two keys, keith and mywife, it has two values which in this case are lists.
to get at the key I can write "for key in dictionary:"I will not get the dict passed as a variable. I would then below have to say dictionary[key] to get at each list in the for loop.
The code below will give me the length of the list ie the number of things in the list that pertains to the key in the dictionary
for key in dictionary:
print(len(dictionary[key]))
To make life easier python lets you call a function on a dictionary, items()
for key, value in dictionary.items:
print(len(value ))
So try this,
def most_classes(teachers):
name_of_busiest_teacher =''
max_classes = 0
for teacher, classes in teachers.items():
if len(classes) > max_classes:
name_of_busiest_teacher = teacher
max_classes = len(classes)
return name_of_busiest_teacher
def num_teachers(teachers):
number_of_teachers = 0
for teacher in teachers:
number_of_teachers += 1
return number_of_teachers
def stats(teachers):
teachers_and_classes_list = []
for teacher, classes in teachers.items():
# so append the list with another list containing the teachers name
# (teacher the key of the dict, and the number(len) of classesfrom the value (classes)
teachers_and_classes_list.append([teacher, len(classes)])
return teachers_and_classes_list
def courses(teachers):
course_list = []
# I'm going to do this a differnt way so you can see dictionary useage without items()
for teacher in teachers:
for course in teachers[teacher]: # see the key in the name of the dict[key] so that lets use iterate over the values.
course_list.append(course)
return course_list

Jan M
1,053 PointsThanks for the detailed answer :) Still don't understand it 100% though:(
In the below code why does python know what I mean by key? I thought that was just a placeholder... Also why does it count the length of the value (a list in this case) if the code says len(dictionary[key])?
for key in dictionary:
print(len(dictionary[key]))
Also, what does the comma in this code tell python to do?
for key, value in dictionary.items:
print(len(value ))
I think I just don`t get the whole loops thing, could you please ellaborate on that?
Sorry for the bunch of stupid
questions, I am just really confused and would really love to understand this stuff :/

David Wright
4,437 PointsHey Jan! Don't fret too much about it. I was in the same boat a day or two ago, but it came full circle for me with the help of the Treehouse Community and several hours focusing on the code. You'll get it!
As for your for loop question: "for key in dictionary: print(len(dictionary[key]))" the len is referencing the values that belong to those [keys] in the dictionary. That's just how the syntax is set up. I agree, it is a little counterintuitive (at least at first).
The dict.items() is a method of dictionaries that, I personally, found to be quite helpful once I found out about it (just yesterday from a MOD on here). The nice thing about it is that it grabs both the key and the value from the dictionary To my understanding, the "key, value" and, the comma, that you ask about are simply the specific syntax setup you use when executing the .items() method on a dict in a for loop (i.e., when your wanting to grab both the key and the values from the dict) I'm confident that this is why python knows what your are talking about when you use the word key in this instance (because it knows you are using a for loop with the .items() method, on a dictionary. So the syntax is rigid yet powerful.
I don't know Kenneth really went over this in the videos yet, or if he did I must have missed it. But a quick google search of .items() method will give info on what it does:
- It returns (or grabs) both the keys and values of a dictionary
- specifically, it returns as list of tuple pairs (i.e. key, value pairs) from a given dictionary (My guess: this is why the comma must be present)
Keep working at it Jan. You'll have your "light-bulb" moments and progress as long as you stick with it! :)
Jan M
1,053 PointsJan M
1,053 PointsAmazin answer! Really helped! Thanks alot :) Could you just maybe ellaborate over this?