Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial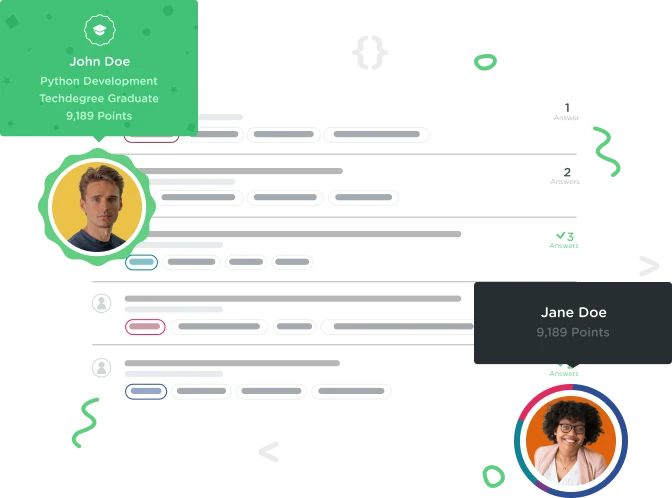
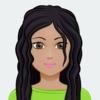
Jeeya M
16,839 PointsRecycler Views: First 3 items getting repeated every 7 items
I made a basic recycler view and it works except that the first 3 items repeat every 7 items. I log to make sure all the data is correct before setting the views and I debug but it hasn't revealed anything. So far I switch my DATA with test data to fill in the list.
My Adapter:
package com.tsm.stormy.adapters;
import android.content.Context;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.tsm.stormy.R;
import com.tsm.stormy.weather.Week;
/**
* Created by Tegh SM on 9/10/2016.
*/
public class DayListAdapter extends RecyclerView.Adapter<DayListAdapter.DayListViewHolder> {
ImageView mIconImageView;
TextView mDayNameLabel;
TextView mTempMaxLabel;
TextView mTempMinLabel;
Week[] mWeek;
public DayListAdapter(Week[] week) {
mWeek = week;
}
@Override
public DayListViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.day_main_list_item, parent, false);
DayListViewHolder viewHolder = new DayListViewHolder(view, parent.getContext());
return viewHolder;
}
@Override
public void onBindViewHolder(DayListViewHolder holder, int position) {
/*if (position < 3) {
holder.bindDayList(mPartialDays[position]);
Log.d("DayList", "mPartialDays");
} else {
//if (position-3 < mWeek.length) {
Log.d("DayList", position + "");
holder.bindDayList(mWeek[position-3]);
//} else {
//Log.d("DayList", "Pos < Week");
//}
}*/
Log.d("DayList", position + "");
holder.bindDayList(mWeek[position]);
}
@Override
public int getItemCount() {
//Log.d("DayList", mWeek.length + " and " + mPartialDays.length);
return mWeek.length;
//+ mPartialDays.length;
}
public class DayListViewHolder extends RecyclerView.ViewHolder {
private Context mContext;
public DayListViewHolder(View itemView, Context context) {
super(itemView);
mContext = context;
mIconImageView = (ImageView) itemView.findViewById(R.id.iconImageView);
mTempMaxLabel = (TextView) itemView.findViewById(R.id.temperatureMaxLabel);
mTempMinLabel = (TextView) itemView.findViewById(R.id.temperatureMinLabel);
mDayNameLabel = (TextView) itemView.findViewById(R.id.dayNameLabel);
}
public void bindDayList(Week week) {
mIconImageView.setImageResource(week.getIconId());
//mTempMinLabel.setText(week.getTemperatureMin(MainActivity.isCel)); // you have to add the param, getters in Week for MinTemp
mTempMaxLabel.setText(week.getTemperature());
Log.d("DayList", week.getLabel() + " : Week");
mDayNameLabel.setText(week.getLabel());
}
}
}
Github project:
https://github.com/tsmadm/Stormy-WeatherApp/tree/V3/app/src/main

Ben Deitch
Treehouse TeacherHey Tegh! I'm not entirely sure what's causing your problem. I do have one thought though - typically we would declare the Views inside the ViewHolder as opposed to inside the adapter. So I'd try moving this bit down into DayListViewHolder.
ImageView mIconImageView;
TextView mDayNameLabel;
TextView mTempMaxLabel;
TextView mTempMinLabel;
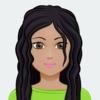
Jeeya M
16,839 PointsBen Deitch Hi thanks, I put the views in the View Holder. Ok so I thought it was caching the values incorrectly, so what I did was I set the cache size so I doesn't repeat.
recyclerView.setItemViewCacheSize(9);
Therefore it was caching and repeating, as the recyclerview generally does, but it was caching incorrectly. If you have a better way to solve this please let me know.
I haver another question also about recycler views here ---> https://teamtreehouse.com/community/is-there-a-way-to-expand-and-show-details-of-recycler-view-items-on-click
Jeeya M
16,839 PointsJeeya M
16,839 PointsBen Jakuben Ben Deitch help please