Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial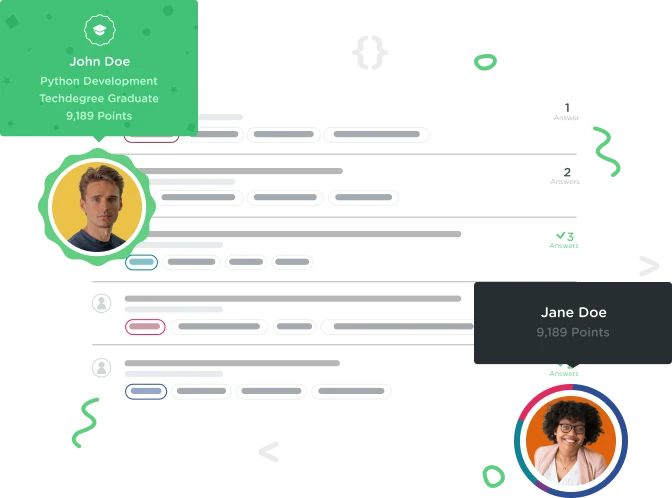

Cesar Garcia
Full Stack JavaScript Techdegree Student 5,184 PointsReduce Method Question
Hey all - I'm not sure what I'm doing wrong here. Every time I run the code, it adds up to 6 instead of 3. What am I missing?
const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"];
let numberOf503;
// numberOf503 should be: 3
// Write your code below
numberOf503 = phoneNumbers.reduce( (count, number) => {
for ( let i = 0; i < phoneNumbers.length; i++ ) {
if ( number[i][1] === '5') {
return count + 1;
}
return count;
}
}, 0);
1 Answer
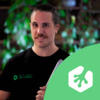
Chris Adams
553 PointsYou're extremely close to the solution here, Cesar Garcia! It's worth taking a deeper look at how the reduce()
method operates. By now you're probably pretty familiar with the forEach()
method - it iterates over every element in an array. You define a function to be executed on each of these elements as they're iterated over.
reduce()
works in a very similar way. This method iterates over every element in an array automatically, but gives us a bit of additional flexibility and functionality in the form of a counter variable. It's really just a value that gets passed to the next iteration, and to the next, and to the next, etc.
So we're looking at a syntax that reads like this:
yourArray.reduce( (previousValue, currentValue) => { /* ..... */ }, initialValue);
Or in your current implementation:
phoneNumbers.reduce( (count, number) => { /* ..... */ }, 0);
count
is the value carried through each iteration (and is eventually returned by the function).
number
is the current value or current index being evaluated within the array.
0
is the initial value that we'll start our count at.
So let's take a look at what you're trying to do inside the function:
for ( let i = 0; i < phoneNumbers.length; i++ ) {
if ( number[i][1] === '5') {
return count + 1;
}
return count;
}
Instead of immediately performing your test on the current number in the phoneNumber
array, you're introducing an unnecessary additional nested for loop
. This means that every index in the initial phoneNumber array runs its own loop*. Hence why your final count is larger than expected.
const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"];
let numberOf503;
// numberOf503 should be: 3
// Write your code below
numberOf503 = phoneNumbers.reduce( (count, number) => {
if ( number[1] === '5') {
return count + 1;
}
return count;
}
}, 0);
If we remove the additional nested loop, you should return the correct result. However, just keep in mind that this solution would tally all numbers starting with a 5, and not just numbers starting with "503" as the instructions suggest.
I'd love to see if you can solve the challenge using an additional method on the String object! https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String