Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial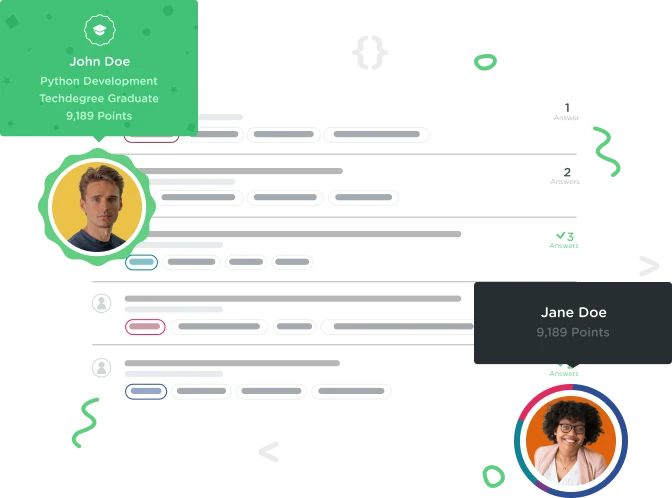

Saloni Mude
9,933 PointsRefactor Challenge, don't understand why function doesn't work
var html = '';
var red;
var green;
var blue;
var rgbColor;
function tenDots() {
for(var i =0 ; i <10; i+=1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
}
document.write(tenDots());
When i run this , instead of having the value of html print out to the document , I just get a message that says "undefined"
5 Answers
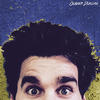
Oliver Duncan
16,642 PointsYour function doesn't return a value, so it returns undefined when called. Try this:
var html = '';
var red;
var green;
var blue;
var rgbColor;
function tenDots() {
for(var i =0 ; i <10; i+=1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
return html;
}
document.write(tenDots());
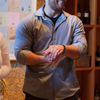
Jonathan Ankiewicz
17,901 Pointsfunction tenDots(a) {
var html = '';
var red;
var green;
var blue;
var rgbColor;
for(var i = 0 ; i < 10; i++) {
red = Math.floor(Math.random() * 255 );
green = Math.floor(Math.random() * 255 );
blue = Math.floor(Math.random() * 255 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="width:20px;height:20px;border-radius:50%;background-color:' + rgbColor + '"></div>';
}
document.body.innerHTML = html;
}
tenDots();
You forgot to declare the size of the div's. You are writing a function to the document, not the actual html. Your calling the function incorrectly as well.
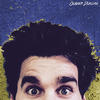
Oliver Duncan
16,642 PointsIf the function returns a value, then writing that function to the document is writing that value (in this case the html string) to the document. His function didn't have a return value (in other words had a return value of undefined), and therefore that's what he was writing to the document. As far as I know, it doesn't have anything to do with specifying the <div> sizes. It's certainly not best practice to write inline css to style your elements.
That said, your function certainly works, it just approaches the problem differently by writing the html string and appending to the document all in one go. But my example, returning a string, will also have the desired effect.
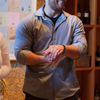
Jonathan Ankiewicz
17,901 PointsOliver Duncan - Did you test your function? I tried your fix before approaching it and it didn't work.
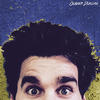
Oliver Duncan
16,642 PointsYeah I tested in Node, with a console.log(tenDots()) instead of a document.write:
> console.log(tenDots());
<div style="background-color:rgb(235,136,162)"></div><div style="background-color:rgb(12,214,142)"></div><div style="background-color:rgb(45,91,193)"></div><div style="background-color:rgb(242,63,211)"></div><div style="background-color:rgb(11,165,21)"></div><div style="background-color:rgb(208,72,53)"></div><div style="background-color:rgb(22,91,251)"></div><div style="background-color:rgb(162,74,22)"></div><div style="background-color:rgb(93,238,138)"></div><div style="background-color:rgb(118,132,225)"></div>
undefined
Ha not the best way to post a console log. But you get the idea. When you call a function that returns a string, it's the same thing as setting a variable to that string and referencing it:
var helloWorld = "Hello World!"
function returnHelloWorld() {
return "Hello World!"
}
helloWorld === returnHelloWorld() // returns true
Perhaps a better way to put it would be to set the function's return value to a variable, then write it to the document that way:
var tenDotsString = tenDots();
document.write(tenDotsString);
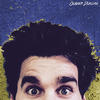
Oliver Duncan
16,642 PointsThat said, I haven't done this exercise/challenge. I assume they would have a separate CSS file that specifies the size of any div on the page, because it really is a good idea to avoid all that inline styling. Especially when presumably we're all learning the basics of JavaScript.
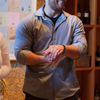
Jonathan Ankiewicz
17,901 Points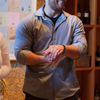
Jonathan Ankiewicz
17,901 PointsIf you saw, he didn't declare a class name on the element.
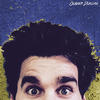
Oliver Duncan
16,642 PointsWe should probably keep our discussion confined to the comments; no need to add extra answers. I went ahead and found the teacher's solution to the problem:
var html = '';
var red;
var green;
var blue;
var rgbColor;
for (var i = 0; i < 10; i += 1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
As I suspected, the project files include a stylesheet that takes care of styling the dots for you. All the student needs to do is write the script - no styling or class names needed. In this case, Dave (the teacher) didn't use a function to create the string, but I hope you can see that document.write(tenDots())
is a valid solution when tenDots() returns a string.
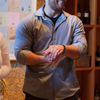
Jonathan Ankiewicz
17,901 Pointsgood point