Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial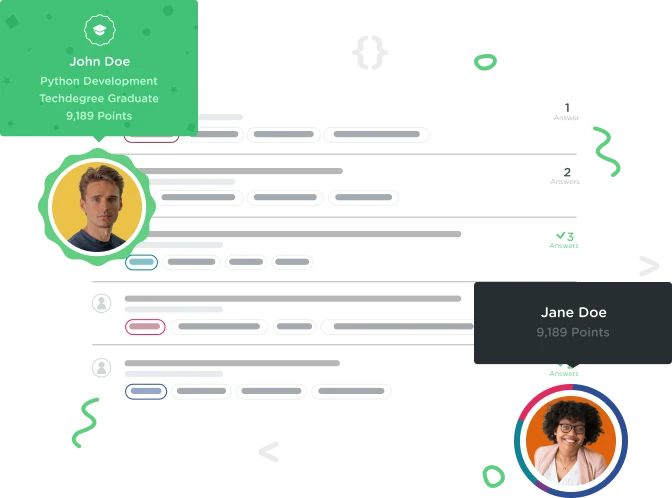

Idris Abdulwahab
Courses Plus Student 2,961 Pointsremoving items from a list
PLEASE, if you are responding to this, do not just give me hints. I got that before and it wasn't really helping due to communication gap perhaps. I need you to help with hints and also adjust the code. That will help me see through the problem and learn better.
Quiz challenge: OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end. I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you! Oh, be sure to look for both uppercase and lowercase vowels!
def disemvowel(word):
base = list(word)
vowels = "aeiou"
small = vowels.lower()
big = vowels.upper()
for char in base:
if char == small or char == big:
base.remove(char)
word = "".join(base)
return word
3 Answers

andren
28,558 PointsThere are two issues with your code:
You check if
char
is equal tosmall
orbig
instead of checking ifchar
is contained insmall
orbig
.You are trying to remove items from the
base
list while you are looping overbase
. Removing items from a list while it is being looped over causes issues since the items end up moving around while the loop is running, which causes some items to be skipped over.
If you change your == comparison to in
comparisons and use the word
variable as the source of your loop instead of the base
variable like this:
def disemvowel(word):
base = list(word)
vowels = "aeiou"
small = vowels.lower()
big = vowels.upper()
for char in word: # Loop over `word` since that will not change during the loop.
if char in small or char in big: # check if `char` is `in` `small` or `big`.
base.remove(char)
word = "".join(base)
return word
Then your code will work.

Idris Abdulwahab
Courses Plus Student 2,961 PointsHi Abubakar,
The .join()
method is used a list to combine the elements in that list into a string.
For example:
boy = "abcd"
girl = list(boy)
wow = "".join(girl)
Details:
boy is a string
girl converts your string into a list like this: ['a', 'b', 'c', 'd']
the .join() is used to combine elements in the list (which is girl) into a string. Now you should have your initial string back which is 'abcd'. Note that before you use the join() method, you have to first create an empty string which is the ""
so that whatever elements being joined together to form a string will be put into that empty string. There has to be a bag before you can put a content into it.
I hope this helps.
Cheers.

Abubakar Gataev
2,226 Pointswhat is the " ".join(base)please answer
Idris Abdulwahab
Courses Plus Student 2,961 PointsIdris Abdulwahab
Courses Plus Student 2,961 PointsThank you so much for your help and hints. I learnt from it and I do appreciate it.