Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial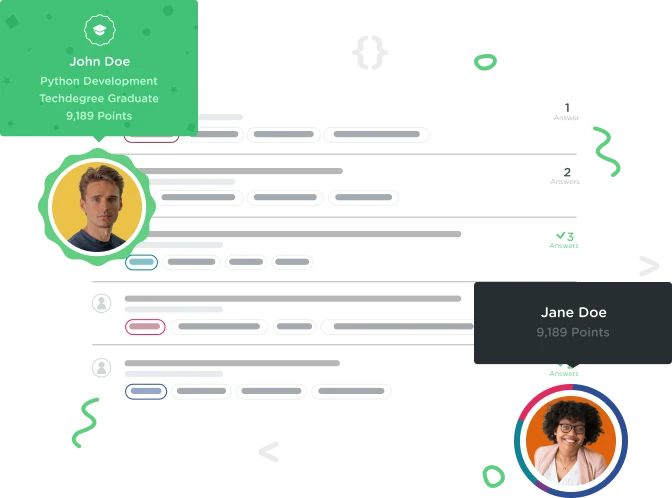
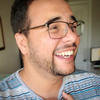
brevans26
15,198 PointsRenaming var rgbColor to var i from the beginning and difference between setting i = 0; i >= 9 and i = 1; i>=10.
Okay! I have two different questions. I understood the for loop concept but I was a little bit intrigued with shortening the variable names to "i". Here is my code:
var html = ' ';
var red;
var green;
var blue;
var i;
for(i = 1; i <= 10; i += 1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
For some reason, it worked. My way of thinking was: 1) Putting the rgbColor inside of the condition like
for (rgbColor = 1; rgbColor >= 10; rgbColor += 1)
2) Then I remember we could short this condition by replacing the name of the variable for "i", so I thought I should go back and rename the rgbColor variable from the beginning.
3) For my final code, I used the rgbColor variable name inside of the loop to define the junction of the red, green, blue variables since, for some reason, using i as a variable name led the browser to behave in a weird way. Check!
var html = '';
var red;
var green;
var blue;
var i;
for (i = 1; i <= 10; i += 1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
i = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + i + '"></div>';
}
document.write(html);
The question is: Why it behaves like that? Isn't "i" a valid name for a variable?
The second (and maybe stupid) question: Is there any difference between the two loops below? 1)
for (i = 1; i <= 10; i += 1) {}
and 2)
for (i = 0; i <= 9; i += 1) {}
Thanks!
Brytner
2 Answers

JEFF CHAI
7,564 Pointsfor (i = 1; i <= 10; i += 1) {
<!-- 'i' is initialize as 1 starting from the for loop -->
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
i = 'rgb(' + red + ',' + green + ',' + blue + ')';
<!-- from above line 'i'is becoming 'rgb(1,2,3)' since you assign the string to 'i' -->
html += '<div style="background-color:' + i + '"></div>';
<!-- At the end of for loop it check condition here again i <= 10
But this time your 'i' is not number anymore, since been assign to string.
-->
}

JEFF CHAI
7,564 Pointsfor (i = 1; i <= 10; i += 1) {}
'i' is just a variable, you can put any variable as you like just to fulfill your requirement. In this line above, is initialise i=1 and when the for loop finish 1 time, it will execute i= i+1. At the end, when i is greater than 10 (execute 10 times), it will break out from the for loop.
If let say, when we change the code as below
for (i = 1; i <= 10; i += 2) {}
Every time the loop go through all the code inside, it will execute i = 1+2
var html = '';
for (i = 1; i <= 10; i += 2) {
html += '<p>Value of i = '+i +'</p>';
}
html += '<p>Leave for loop</p>';
<!--Result
Value of i = 1
Value of i = 3
Value of i = 5
Value of i = 7
Value of i = 9
Leave for loop
-->
Okay, now back to your question,
i = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + i + '"></div>';
In this line, you assign 'i' become a string like 'rgb(1,2,3)' and next line with add a div into html. Come to this part still don't have any error yet. After this line, for loop will start to check condition, i <=10 in here will become 'rgb(1,2,3)' <=10 . At this point your script will give you error, because you are comparison string with the integer.
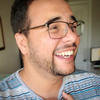
brevans26
15,198 PointsBut this is the thing: the console does not spit out any errors in both codes. About "i" becoming a string, how is that possible? i and rgbColor are just names I chose for the variable. Sorry, but I did not understand this last part of your explanation.
brevans26
15,198 Pointsbrevans26
15,198 PointsIt makes sense now after a good night of sleep and a cup of coffee! Haha!
Thanks JEFF CHAI !