Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial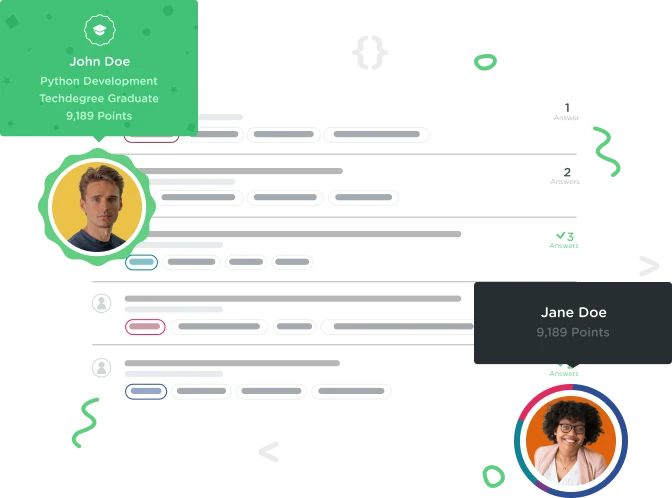

dlpuxdzztg
8,243 Points'requestFeature() must be called before adding content'
Hello.
When I run my app and open up a DialogFragment, the app crashes and I'm given this error:
12-13 10:12:00.291 25576-25576/com.x.quicklist E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.x.quicklist, PID: 25576
android.util.AndroidRuntimeException: requestFeature() must be called before adding content
at com.android.internal.policy.impl.PhoneWindow.requestFeature(PhoneWindow.java:325)
at com.android.internal.app.AlertController.installContent(AlertController.java:231)
at android.app.AlertDialog.onCreate(AlertDialog.java:356)
at android.app.Dialog.dispatchOnCreate(Dialog.java:406)
at android.app.Dialog.show(Dialog.java:279)
at android.app.DialogFragment.onStart(DialogFragment.java:496)
at android.app.Fragment.performStart(Fragment.java:2086)
at android.app.FragmentManagerImpl.moveToState(FragmentManager.java:922)
at android.app.FragmentManagerImpl.moveToState(FragmentManager.java:1067)
at android.app.BackStackRecord.run(BackStackRecord.java:833)
at android.app.FragmentManagerImpl.execPendingActions(FragmentManager.java:1452)
at android.app.FragmentManagerImpl$1.run(FragmentManager.java:447)
at android.os.Handler.handleCallback(Handler.java:739)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:135)
at android.app.ActivityThread.main(ActivityThread.java:5351)
at java.lang.reflect.Method.invoke(Native Method)
at java.lang.reflect.Method.invoke(Method.java:372)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:908)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:703)
While the error doesn't explain where the error is located in my code, I do believe it has something to do with my DialogFragment:
private TextView mYesTextView;
private TextView mNoTextView;
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context);
View view = getActivity().getLayoutInflater().inflate(R.layout.dialog_fragment_prompt, null);
AlertDialog dialog = builder.create();
builder.setView(view);
dialog.setContentView(R.layout.dialog_fragment_prompt);
dialog.getWindow().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT));
mYesTextView = (TextView) view.findViewById(R.id.yesTextView);
mNoTextView = (TextView) view.findViewById(R.id.noTextView);
return dialog;
}
2 Answers

Seth Kroger
56,413 PointsWhenever you're using a Builder pattern, builder.create() or builder.build() should be the final method called on it. Doing additional setup steps to the builder afterward don't help because the object is already built and you should either move those steps to occur beforehand or work on the built object.
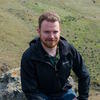
David Remington
18,326 PointsTry reversing these two lines:
AlertDialog dialog = builder.create();
builder.setView(view);
I have a hunch that the dialog is being built before it knows what resources to use while inflating it.