Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial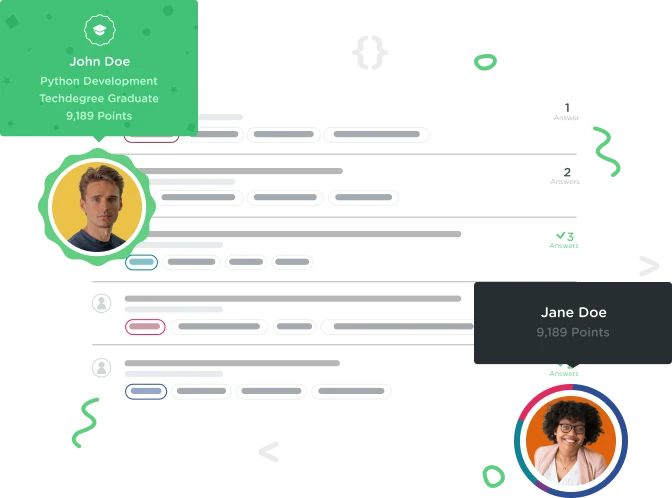

Zan Le
1,016 PointsReturn a list of list from dictionary
Hi, in the stats function, after I create a new dictionary, and then convert it to a list of list using map(list, ...), it shows me the desired output. however, it does not pass the test. could you kindly help me on this please.
many thanks
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(string):
return len(string)
def num_courses(string):
course_list=[]
for x in string.values():
for y in x:
if y not in course_list:
course_list.append(y)
return len(course_list)
def courses(string):
course_list=[]
for x in string.values():
for y in x:
if y not in course_list:
course_list.append(y)
return course_list
def most_courses(string):
maxcount = max(len(value) for value in string.values())
return "".join([k for k, value in string.items() if len(value) == maxcount])
def stats(string):
new_dict = {k: len(v) for k,v in dict.items()}
new_list = map(list, new_dict.items())
return new_list
2 Answers

Krishna Pratap Chouhan
15,203 PointsHINT: You missed to use "string" variable that you were taking as an argument for stats function.
solution is here:
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(string):
return len(string)
def num_courses(string):
course_list=[]
for x in string.values():
for y in x:
if y not in course_list:
course_list.append(y)
return len(course_list)
def courses(string):
course_list=[]
for x in string.values():
for y in x:
if y not in course_list:
course_list.append(y)
return course_list
def most_courses(string):
maxcount = max(len(value) for value in string.values())
return "".join([k for k, value in string.items() if len(value) == maxcount])
def stats(dict):
new_dict = {k: len(v) for k,v in dict.items()}
new_list = map(list, new_dict.items())
return new_list
btw its not a good idea to use the variable name as "dict".

Zan Le
1,016 PointsThank you!

Krishna Pratap Chouhan
15,203 PointsTodd Costa , it takes time... You see enough of it and you start understanding it eventually. I can recall that i couldn't understand the above piece of code a while back and i struggle to understand a lot of it even now. Dont worry about that, at all.
In fact i believe, a code is good when anyone can understand it. Even those who don't understand that language should be able to make sense out of it.
Josh Bennett
15,258 PointsJosh Bennett
15,258 PointsWhat is going on here?
.join([k for k,
I get everything else.
Krishna Pratap Chouhan
15,203 PointsKrishna Pratap Chouhan
15,203 Pointswill give the output, 'one-and-two-and-three'.
so there is join function.
Quite concise and good code by Zan Le.
Todd Costa
3,380 PointsTodd Costa
3,380 PointsSort of an unrelated question and i understand that everyone learns at their own pace, but am i behind because i dont even think or understand how to write code that concise. I thought i was pretty good at parsing others code but the above code might as well be binary to me.