Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial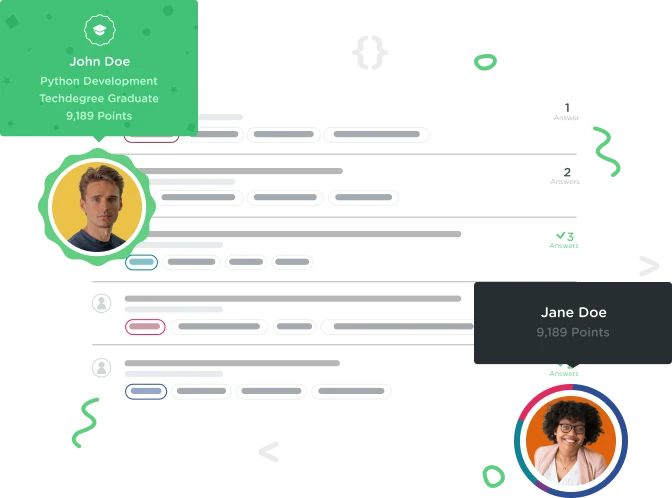

Manish Giri
16,266 PointsReturn dictionary of word count is not accepted
The question asks to create and return a dict
where the keys would be each of the words in the string argument, and the values would be a count of how many times each word appears in the string.
Somehow, my code isn't accepted. I've checked on repl.it, the output looks the same as what is expected. Here's the repl.it link - https://repl.it/Nu8v/0
I'm using List Comprehension to split the argument into string (on whitespace), and then construct the dict
.
My code is attached below -
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(words):
return {s.lower(): words.count(s) for s in words.split(" ")}

Manish Giri
16,266 PointsHi,
Thank You for pointing out my mistake! Just realized that .count()
works differently on strings than on lists, which is why it wouldn't work.
I got it working using List Comprehension, just had to create a list first, as for Lists, .count()
would count only how many times the item occured as an individual element of the list.
Here's the fixed code -
def word_count(s):
words = s.lower().split()
return {item: words.count(item) for item in words}
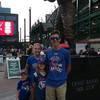
AJ Salmon
5,675 PointsHappy to help! Thanks for your explanation as well.
2 Answers
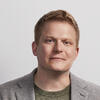
Keith Whatling
17,759 PointsYour words.count(s) is counting all the letters in a word, not the individual word. Its ugly but this was my solution. I think you need to refer to the dict as you are constructing it, not sure how to do that.
def word_count(words):
words = words.lower().split(' ')
toReturn = dict()
for word in words:
if word in toReturn.keys():
toReturn[word] += 1
else:
toReturn.update({word : 1})
return toReturn
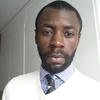
Marcus Sassi
4,394 PointsThis should do it
myword ="I do not like it Sam I Am"
def word_count(sentence):
my_dict = {}
sentence = sentence.lower().split()
for sen in sentence:
if sen in my_dict:
count = my_dict[sen] + 1
my_dict[sen] = count
else:
my_dict[sen] = 1
return my_dict
AJ Salmon
5,675 PointsAJ Salmon
5,675 PointsI don't know much about list comprehension yet, but after playing around with your code, it looks like if a letter sequence is a stand-alone word and is also contained in another word, it counts the sequence every time it appears. For example, 'An anaconda and a dog" returns {'an': 1, 'anaconda': 1, 'and': 1, 'a': 5, 'dog': 1}. Notice that 'a' is counted 5 times, when it only actually appears once as an independent word. Like I said, I'm not very familiar with list comprehension, so I can't really offer a solution here, but that's a possible issue that I noticed. Hopefully it's helpful in some small way. If not, I tried, haha. I really like the idea of list comprehension, so I'm eager to see what the solution to this one is. Good luck!