Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial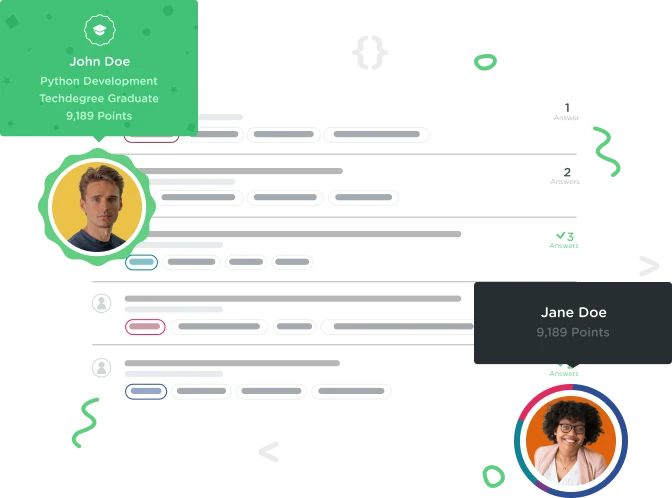

vsiddharthverma
3,182 Pointsreturn not and while
import random start = 5 def even_odd(num): # If % 2 is 0, the number is even. # Since 0 is falsey, we have to invert it with not. return not num % 2 while start: a = random.randint(1,99) if even_odd(a): print ("{} is even".format(a)) else: print("{} is odd".format(a)) start-=1
I do not totally understand the meaning of return not num%2. num%2 means yields nothing? Also for while start: , if start = -1, while will return false? and the loop will stop?
Anybody can help answer? Thanks a lot!
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
a = random.randint(1,99)
if even_odd(a):
print ("{} is even".format(a))
else:
print("{} is odd".format(a))
start-=1
2 Answers
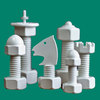
Steven Parker
231,269 Points
It may seem confusing to treat numbers as "true" and "false" (but it's very handy!).
The concept of "truthy" and "falsey" is that ordinary numbers can be treated as if they are "true" or "false" based on their value. Just remember that 0 is "falsey" (false), and all other numbers are "truthy" (true).
So "num % 2
" is a remainder function. The remainder of dividing any even number by 2 is 0, and any odd number is 1. Putting "not
" in front of it means to treat the value as a true/false, which means 1 will be "true" and 0 will be "false". But "not
" also means to take the opposite, so anything that was true before (1) will now be "false", and anything that was false (0) will now be true. So the whole thing returns "true" if the number is even, and "false" if it is odd. The challenge calls this function "even_odd", but I think a better name might be "is_number_even".
And since 0 is "falsey", the while will consider start to be false when it is 0, and the loop will stop.

vsiddharthverma
3,182 PointsThank you Steve! Very helpful!