Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial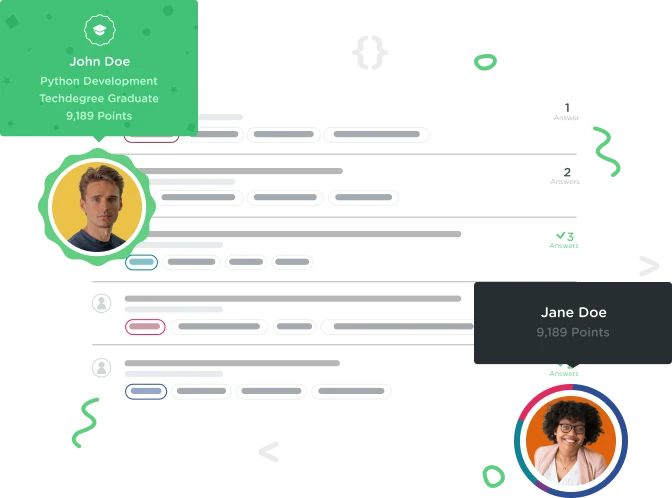
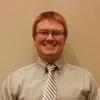
Patrick Shushereba
10,911 PointsReturning the correct index in an array.
I'm trying to write this method, and the error that I get is that the find_index method is not returning the correct index. I tried using return index(name) but that didn't help me either.
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
index = 0
found = false
if todo_items.include?(name)
return index
else
return nil
end
index += 1
end
end
2 Answers

Amy Kang
17,188 Pointsdef find_index(name)
# this is the starting index of the array
index = 0
found = false
# Here you are iterating through each item in the array
todo_items.each do |item|
# if the item is found, found variable is set to true
found = true if item.name == name
# This will stop the .each method if found is set to true
break if found
# otherwise the index will increase as you move on to the next item in the array
index += 1
end
# if the item is found, it will return the index, otherwise it will return nil
if found
return index
else
return nil
end
end
Also, the method is checking item.name against name because the name parameter being passed into the method is a string. If you just check it against the item object it will return false because the string and item object are not equal.
In order to check the item name against a string, you must specify which part of it you want to check. In this case you want to the check item's name against the name you are searching for.
Basically, item itself is an object, the name argument passed to the method is not. Therefore you must specify exactly what inside the object you are looking for: item.name
Hope that helps someone!
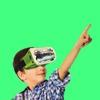
John Hill
Front End Web Development Techdegree Graduate 35,236 PointsHi there, this code comes from another forum thread, so I can't help explain why it works, but I tested it and it completes the challenge. Maybe you can look at it and see why it works, or someone else more fluent in Ruby can explain the details.
def find_index(name)
index = 0
found = false
todo_items.each do |item|
found = true if item.name == name
break if found
index += 1
end
if found
return index
else
return nil
end
end
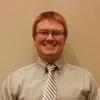
Patrick Shushereba
10,911 PointsI follow what's going on in a broad sense. My question is why you check if item.name = name rather than just item by itself. If someone could clear that up I'd appreciate it.