Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial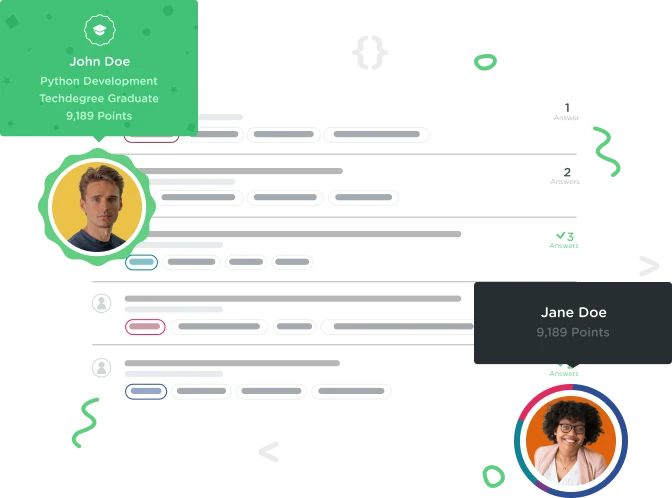
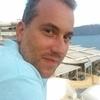
yannis sousanis
7,199 Pointsreverse evens issue
the def reverse_evens(a) runs perfectly on my ide but not here I used this: return a[len(a)::-2]
def first_4(a):
return a[0:4]
def first_and_last_4(a):
return a[0:4] + a[-4:len(a)]
def odds(a):
return a[1::2]
def reverse_evens(a):
return a[len(a)::2]
2 Answers
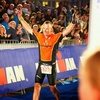
Steve Hunter
57,712 PointsHi Yannis,
The comment to your answer hasn't quite got the right process, in that they have it backwards. You want to get the evens first (store that in a local variable), then reverse & return that. If you do this the other way round, the output varies depending on the length of the iterable; it'll only be correct half the time.
You can also tidy up your first_and_last_4
method too. There's no need to check the length of the iterable; just start at -4 and go to the end for the last_4
part; for the first_
part, just start at the beginning and go to 4, like this:
def first_and_last_4(a):
return a[:4] + a[-4:]
def reverse_evens(a):
evens = a[::2]
return evens[::-1]
I hope that helps.
Steve.
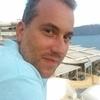
yannis sousanis
7,199 PointsThank you for your answer may I ask something? My question was different, on my computer I used
def reverse_evens(a):
return a[len(a)::-2]
When i made the question i had put this piece of code in but for some reason it uploaded the old one. what do I do wrong in this function?
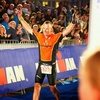
Steve Hunter
57,712 PointsHi Yannis,
We want a solution that returns [5, 3, 1]
from [1, 2, 3, 4, 5]
and it should return [5, 3, 1,]
from [1, 2, 3, 4, 5, 6]
, right?
If you take both these inputs with your method, and substitute the length of each list, we get:
# for [1, 2, 3, 4, 5] input; 5-long
def reverse_evens(a):
return a[5::-2]
# for [1, 2, 3, 4, 5, 6] input; 6-long
def reverse_evens(a):
return a[6::-2]
I think we start counting at zero for lists, i.e. the first element is index 0, the last index is either 4 or 5, depending which input list we use. So using len(a)
gives too high a starting position as that index is out of bounds. Let's assume that the compiler can handle that and it just starts from the end of the list, whatever its index is.
The first example would return what we expect, I think, it will start at the last element and skip over the remaining elements, adding every other one to the returned list. So, we get [5, 3, 1,]
- lovely! What do we get with the second input list which has an even number of elements? We'd get [6, 4, 2]
which isn't what we want.
So trying to reverse the list first then select evens on the reversed list gives a length-dependent output, which the challenge is not looking for.
Make sense?
Steve.
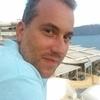
yannis sousanis
7,199 PointsGot it thank you very much. Thanks for your help
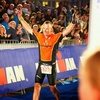
Steve Hunter
57,712 PointsNo problem - glad to help. :+1;
Ismail KOÇ
1,748 PointsIsmail KOÇ
1,748 Pointsin this function, you have to make first reverse list later return slice with evens because python starts last character of list
alternatively you can use that