Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial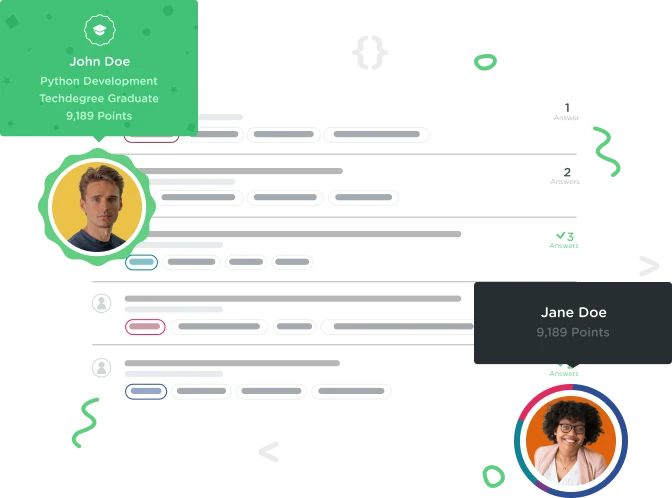

seantipton
7,743 PointsRuby Grocery List Program Question
I built out the grocery list program in the Ruby Collections section and I don't understand how Ruby knows that I want to assign 'item_name' and 'quantity' variables to the key/value pair in the array created in the hash, which is associated with the "items" hash key.
In the 'add_list_item' method created, I reference the hash created in the 'create_list' method but I don't explicitly assign the 'item_name' and 'quantity' variables/hash values to the "items" hash key. The code in the 'add_list_item' reads, "hash = {"name" => item_name, "quantity" => quantity}". How does ruby know that the hash value I am referring to is associated with the "item" hash key created in the 'create_list' method?
Does Ruby implicitly associate the 'item_name' and 'quantity' variable values to the hash key "items", based on the fact that it is the only empty hash value in the 'hash' hash?
I hope the above makes sense. I am new to Ruby and programming in general. Any info here would be much appreciated. Below is the program code in question.
def create_list
print "What is the name of your list? "
name = gets.chomp
hash = { "name" => name, "items" => Array.new}
end
def add_list_item
print "What is the item called? "
item_name = gets.chomp
print "How much? "
quantity = gets.chomp.to_i
hash = {"name" => item_name, "quantity" => quantity}
return hash
end
def print_separator(character = "-")
puts character * 80
end
def number_of_items
puts "How many items would you like to add? "
num_items = gets.chomp.to_i
return num_items
end
def print_list (list)
puts "list: #{list["name"]}"
print_separator()
list["items"].each do |item|
puts "\tItem: " + item["name"] + "\t\t\t" + "Quantity: " + item["quantity"].to_s
end
print_separator()
end
list = create_list()
num = number_of_items()
num.times {list["items"].push(add_list_item())}
print_list(list)
p list
1 Answer

Joe Purdy
23,237 PointsDoes Ruby implicitly associate the 'item_name' and 'quantity' variable values to the hash key "items", based on the fact that it is the only empty hash value in the 'hash' hash?
Ruby doesn't implicitly assign the returned hash from the add_list_item
method to the "items" key in your list hash. Instead you're telling Ruby to push a new hash value into the "items" array value. Take a look at these sections of your program:
def create_list
print "What is the name of your list? "
name = gets.chomp
hash = { "name" => name, "items" => Array.new}
end
...
list = create_list()
num = number_of_items()
num.times {list["items"].push(add_list_item())}
In the first snippet is your create_list
method which returns a new hash with a "name" key assigned to the name provided by user input. The other key in this hash, "items", is initialized as an empty array value.
The second snippet shows where you create a new variable named list
which initializes using the create_list
method as a list hash. You're adding new list items by calling list["items"].push(add_list_item())
which uses the Array.push
method to append a new hash value returned from the add_list_item
method into the list["items"]
array.
I hope that helps clear up what Ruby is doing here. Because the data type of the "items" key in the list hash is an array you can simply use array methods like push to append new array values. In this case the data value being pushed to the items array is a new hash with keys for the item name
and quantity
.