Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial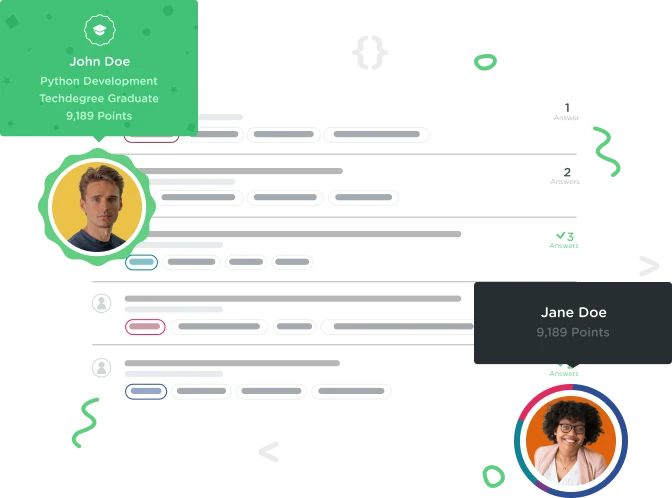

dylan kane
2,772 Pointsruby on rails static pages
whats the point of static pages? for example, would it be better to use a login page on a static page or the alternative?

dylan kane
2,772 Pointsyes that helped very much thank you! i also have another question. i am making a ruby on rails web application, and before i even started the rails project, i designed the whole thing with html css and java. now, all i need to do is add sign in sign up and post blog stuff. to do that i need to put the website that is already made into a rails project, what changes would need to be made to the html to make it work exactly like it did when it wasnt in the rails application? if you dont know that is fine
2 Answers
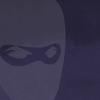
Brenna Holmes
17,629 PointsOk so you want to convert your current site into a rails application and then add some blog functionality? That's an excellent place to start, especially since you have a stake in how the site turns out so you won't abandon the project.
The way I converted mine into a rails project was, for the static stuff, I "rails new appname"'d and "rails generate controller home". That sets up a home directory in views for your pages to go into, the controller you can just add methods like:
def index
end
def projects
end
and use the above routes configuration to make those link to your pages.
Putting your assets into the rails pipeline is a little more complicated, but it's mostly intuitive and rails provides really useful error messages.
I'll just give you 3 tips:
Use Pow - the zero-configuration rack server for rails and the Powder gem so you can "powder open" and it'll launch your project locally.
Install the better errors gem globally. Better error messages are always nice.
-
For images I was stuck on this for a long time, but you need to do this:
<%= image_tag "folder(images is implicit)/image_name.png", :alt => "alt-text", :class => "your_class" %>
That should get you going pretty well :] mark my answers as good/best for me and post a reply if you get stuck, helping people helps me learn too.
Ok last thing, SCSS is standard for rails, so change your css to scss (you can use the same code, just rename your files to mycss.scss) and put them in assets/stylesheets and rails should take care of the rest.
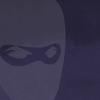
Brenna Holmes
17,629 PointsRemember for linking around your static pages, the better way to start with the controller code above is this:
(YourStaticPageControllerName)Controller.action_methods.each do |action|
get "/#{action}", to: "(YourStaticPageFolder)##{action}", as: "#{action}_page"
end
Then you do this:
<%= link_to 'My Awesome Page', awesome_page_path %>
remember that you have to, with the above code, do yourpagename_page_path.
Alright that's enough to get you going for now.

dylan kane
2,772 PointsYou are the best human being i have even come in contact with, great answer thanks man!!!!!!!!
Brenna Holmes
17,629 PointsBrenna Holmes
17,629 PointsLet's say you want to have informational content that won't change often, a company bio, an overview of your workflow, lists of clients that have used your services, etc.
These pages don't need interactivity, they need only the most basic controllers and views to render, it's a matter of simplicity for me, since the default action is to render the name of the function in a controller, I use this:
to simply name methods after the page names for static pages, and immediately everything works. Static pages are lightweight and they serve a useful purpose, although you're not building a Rails app to host static pages, they have their place.
Did that help at all?