Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial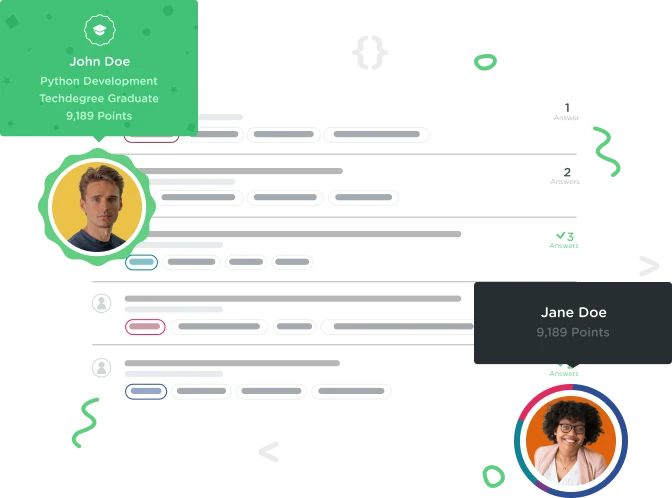
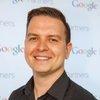
Tim Dlugosz
2,025 PointsScissors, Paper, Rock Game - Is this the code efficient?
Hi I just wanted to see if this code is correct/efficient it seems to work (yay!) but I want to make sure that I'm not making it more cumbersome than it need to be (lots of IF statements). Also is there a way to stop my "puts" statements from dropping on to a new line after I drop in a variable like "#{name}"?
Anyway here is the code:
#Greeting - greets the player and gets their name.
def player
puts "Enter player name: "
name = gets
puts "Hi #{name}Lets play scissors, paper, rock."
return name
end
#Weapon Selection - gets the players weapon of choice.
def select_weapon
puts "Please select your weapon of choice by type: 1 for scissors, 2 for paper or 3 for rock: "
weapon = gets.to_i
if weapon == 1
return "scissors"
elsif weapon == 2
return "paper"
elsif weapon == 3
return "rock"
else
return "error"
end
end
#AI Weapon - the computer picks a weapon.
def ai_select_weapon
aispr = rand(1..3)
if aispr == 1
return "scissors"
elsif aispr == 2
return "paper"
elsif aispr == 3
return "rock"
end
end
#Play! - Runs the game.
name = player
weapon = select_weapon
aispr = ai_select_weapon
puts "You have selected #{weapon}"
puts "The hivemind has selected #{aispr}"
puts "#{weapon} vs #{aispr}"
if weapon == "scissors" and aispr == "paper"
puts "#{name} WINS a cutting victory!"
elsif weapon == "paper" and aispr == "rock"
puts "#{name} surrounds the enemy for the WIN"
elsif weapon == "rock" and aispr == "scissors"
puts "SMASHING! #{name} takes the WIN"
elsif weapon.eql?(aispr)
puts "#{name} and the computer tied. How boring, try again"
else puts "You lose. Try again"
end
1 Answer

Luke Pettway
16,593 PointsYou might benefit from refactoring with a Case statement, but it isn't so cumbersome yet as to really make that much of a difference. http://www.techotopia.com/index.php/The_Ruby_case_Statement here is a link to an article about using case in Ruby.
You need to use .chomp at the end of your gets so that it removes new line characters (automatically added in).
name = gets.chomp
puts "Hi #{name} Lets play scissors, paper, rock."
The above shouldn't return to a new line after #{name}.
Andrew Stelmach
12,583 PointsAndrew Stelmach
12,583 PointsIs it just how you have pasted your code here, or does your code really lack any indentation? If so, you really need to sort that out and get into the habit of indenting properly - it's really hard to read as it is here.