Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial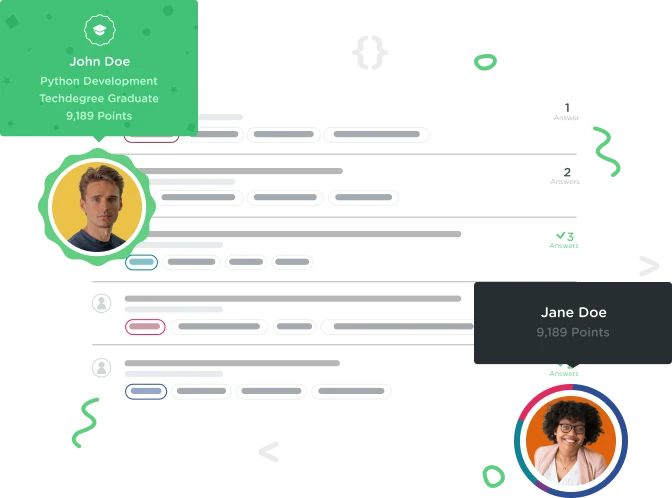

Arkitect 0
12,065 PointsSectioning of Data
Am a bit confused how Pasan sorted out his contact list? Can someone please help
1 Answer
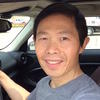
David Lin
35,864 PointsYeah, there's a lot going on in those few lines:
static var sectionedContacts: [[Contact]] {
return sortedUniqueFirstLetters.map { firstLetter in
let filteredContacts = contacts.filter { $0.firstLetterForSort == firstLetter }
return filteredContacts.sorted(by: { $0.firstName < $0.firstName} }
}
}
Take the example:
contacts = ["Dan", "Bob", "April", "Cathy", "Abe"]
... where I'm representing each Contact object by a string which is the Contact's first name for simplicity ... in actuality, it would be Contact(firstName = "Dan", lastName = "Smith", etc ...) for each element.
Then, sortedUniqueFirstLetters is an array of sorted first letters of contacts. So, it would be ["A", "B", "C"].
Now, we are going to apply the .map transformation on sortedUniqueFirstLetters, that is, for every element in the array, we are going to apply the function in the closure.
For the first element in sortedUniqueFirstLetters, which is "A" (assigned to the local variable "firstLetter"), we apply the filter transformation on the contacts array, where we filter on the condition defined in the closure that the first letter of each Contact equals whatever firstLetter is (in this case, "A"). For "A", the elements which satisfy this condition are "April" and "Abe", so the local variable filteredContacts is now ["April", "Abe"]. Finally, we sort this array based on first name in ascending order (as defined in the closure passed into .sorted), so the resulting array which we return is ["Abe", "April"].
So, so far, our return array has one element, and it is [ ["Abe", "April"]].
Likewise, we traverse through all the elements in sortedUniqueFirstLetters, "B" creating the resulting array ["Bob"], "C", ["Cathy"], "D", ["Dan"] ... and so the final array of arrays which is returned as the variable sectionedContacts is:
[["Abe", "April"], ["Bob"], ["Cathy"], ["Dan"]]
Henry Cooper
iOS Development with Swift Techdegree Student 6,182 PointsHenry Cooper
iOS Development with Swift Techdegree Student 6,182 PointsThis was really helpful, thank you David.
Armend Azemi
27,921 PointsArmend Azemi
27,921 PointsI have a question. The second return type in this code, is that returning the local var "filteredContacts" to the first return type?
And you said:
But the return type is [[Contact]] so it's expecting us to return a single nested array, but it looks like we are returning several arrays? We are not "storing" these arrays in any temp [[]] array to return.
I hope my question is clear enough for you to understand.