Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial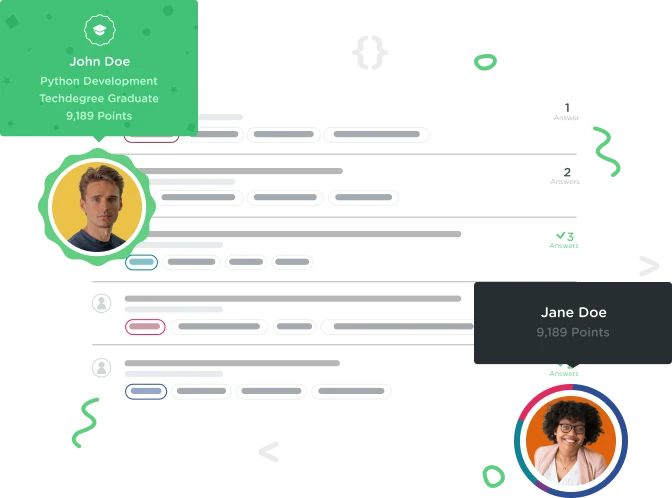
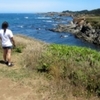
Nancy Melucci
Courses Plus Student 36,143 PointsSending edittext to textview in new activity...
I am writing an app that takes fill in the blank answers for a mad lib using edit texts and sends it to a new activity to create the final version of the story (a limerick, actually, the professor's idea). The program runs and the second activity is called but the text for the final version does not show. I will show the java and xml below. If anyone can help I would be so grateful...
First the java Main and Second Activity, then the XML that goes with them...
//Main Activity
package com.nancy.example.madlibericks;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
public final static String EXTRA_MESSAGE = "com.nancy.example.madlibericks.MESSAGE";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
public void sendMessage() {
EditText editText1 = (EditText) findViewById(R.id.auview1);
EditText editText2 = (EditText) findViewById(R.id.auview2);
EditText editText3 = (EditText) findViewById(R.id.auview3);
EditText editText4 = (EditText) findViewById(R.id.auview4);
EditText editText5 = (EditText) findViewById(R.id.auview5);
Intent intent = new Intent(this, DisplayLiberickActivity.class);
String message = String.format("There once was a %s named Jimmy, who %s, %s on a plane to SimCity, he %s in a train, " +
"and said 'What a pain! I'm %s no more for a day!", editText1, editText2, editText3, editText4, editText5);
intent.putExtra(EXTRA_MESSAGE, message);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
Button msgButton = (Button) findViewById(R.id.btnMake);
assert msgButton != null;
msgButton.setOnClickListener(new OnClickListener() {
public void onClick(View view) {
Toast.makeText(MainActivity.this, "Button Clicked", Toast.LENGTH_SHORT).show();
sendMessage();
}
});
return true;
}
//Second Activity
//show limerick (Second Activity)
package com.nancy.example.madlibericks;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.support.v4.app.NavUtils;
import android.view.MenuItem;
import android.widget.TextView;
// Created by Nancy on 11/25/2016.
public class DisplayLiberickActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.display_finishedliberick);
//get message from the intent
Intent intent = new Intent(DisplayLiberickActivity.this, MainActivity.class);
String message = intent.getStringExtra(MainActivity.EXTRA_MESSAGE);
//create the text view
TextView textView = (TextView)findViewById(R.id.textView);
textView.setTextSize(30);
textView.setText((getIntent().getStringExtra(message)));
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
// This ID represents the Home or Up button. In the case of this
// activity, the Up button is shown. Use NavUtils to allow users
// to navigate up one level in the application structure. For
// more details, see the Navigation pattern on Android Design:
//
// http://developer.android.com/design/patterns/navigation.html#up-vs-back
//
NavUtils.navigateUpFromSameTask(this);
return true;
}
return super.onOptionsItemSelected(item);
}
}
3 Answers
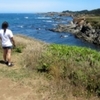
Nancy Melucci
Courses Plus Student 36,143 PointsHere is the XML for the second activity (the one that should show the completed limerick)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:background="@drawable/limerickgradient"
tools:context="com.nancy.example.madlibericks.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceLarge"
android:text="Howdy"
android:id="@+id/textView" />
</LinearLayout>

Seth Kroger
56,413 PointsYou can't concatenate an EditText to a String directly. For each EditText you need to use editText.getText().toString() to get in input value.
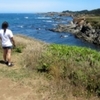
Nancy Melucci
Courses Plus Student 36,143 PointsThat helped but apparently I also needed a bundle message. I added that. The message passes but the editTexts aren't going with it. I'll keep working on it.
Nancy Melucci
Courses Plus Student 36,143 PointsNancy Melucci
Courses Plus Student 36,143 PointsHere is the XML for the main activity