Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial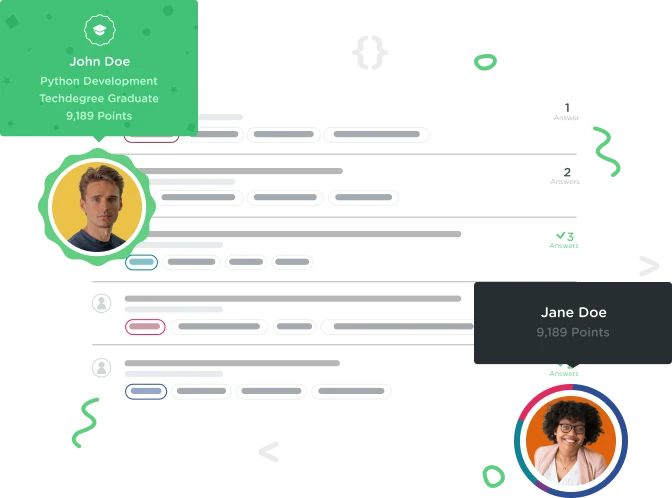

Martin Erlic
58 PointssetOnItemClickListener within Custom Adapter class?
I've tried setting an OnItemClickListener within the View method of my custom Adapter class. Then I moved it into my Activity as per somebody's suggestion:
ListView listView = (ListView)findViewById(android.R.id.list);
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(NotificationsActivity.this, FeedActivitySingle.class);
intent.putExtra(FeedActivitySingle.EXTRA_DESIGN, notifications.getString(ParseConstants.KEY_SENDER_FEED_OBJECT_ID));
startActivity(intent);
}
});
Why doesn't this work? Somebody suggested I set it in the Activity I in which the layout was set up, which in this case would be the NotificationsActivity (but as you can see above, I then can't access notifications.getString to retrieve my Parse Constant). What should I do? I basically want to start an intent whenever a user clicks on a listview item.
For context, I've adapted this tutorial to build an activity which shows all your recent notifications. So I want to launch a new activity whenever a listview item is clicked, and not just when clicking a holder for an image or textview.

Martin Erlic
58 PointsHey Harry James. Thanks for the quick reply!
Well, I'm unable to access the notifications variable (formerly messages) when I move the onItemClickListener into the NotificationsActivity (formerly InboxFragment).
I suppose that's because it's not set in my NotificationsActivity, so how would I do that if I define it in my Adapter class as such?:
public NotificationsAdapter(Context context, List<ParseObject> notifications) {
super(context, R.layout.notifications_listview_item, notifications);
mContext = context;
mNotifications = notifications;
}
Edit: Alternatively, how can I just set an onItemClickListener inside my custom Adapter class? Presently, I can only click on holders. How about clicking on the listview item itself?
2 Answers
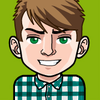
Harry James
14,780 PointsHey again Martin!
What you could do is set up a getter for your notifications in the adapter, as such:
public List<ParseObject> getNotifications() {
return mNotifications;
}
Then, you can access the getNotifications()
method wherever you have a reference to the NotificationsAdapter (That's when you do something like NotificationsAdapter adapter = new NotificationsAdapter(arg1, arg2) like so:
List<ParseObject> notifications = adapter.getNotifications();
This would be written in your onItemClick() method, so that you can get the notifications every time an item is clicked.
Also, you said that you want to launch a new activity afterwards. If you want this activity to have a reference to these notifications, you have two options. You could again do the same and get a reference to the NotificationsAdapter in the new activity or, you could pass the notifications as an extra in the Intent. Note that the passing through the Intent bit is slightly trickier so let me know if this is the route you want to take and I'll help you out with some code :)
Also if you need anything else, I'll get back to you tomorrow :)

Martin Erlic
58 PointsHmm... I'm still a little confused but moving in the right direction I think. Thanks.
I've set an set an OnItemClickListener in my main NotificationsActivity, which is where the Adapter layout is set up. I tested with a Toast and it works! So I can click on things and that's great.
The problem is the following line: intent.putExtra(FeedActivitySingle.EXTRA_DESIGN, notifications.getString(ParseConstants.KEY_SENDER_FEED_OBJECT_ID));
All the intent does is open a new activity with a particular string. There's nothing tricky about this code and it works perfectly when I paste it into my NotificationsAdapter class.
However, when I place this line in my NotificationsActivity the "getString" method cannot be resolved. It's red.
I tried adding the Getter like you suggested but it doesn't seem to be working either way!

Martin Erlic
58 PointsAnother way to do it would be to set the OnClickListener to the convertView! This one actually worked. I placed this in the getView method of my NotificationsActivity.
convertView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent= new Intent(mContext,FeedActivitySingle.class);
intent.putExtra(FeedActivitySingle.EXTRA_DESIGN, notifications.getString(ParseConstants.KEY_SENDER_FEED_OBJECT_ID));
mContext.startActivity(intent);
}
});
Harry James
14,780 PointsHarry James
14,780 PointsHey Martin!
Just wanted to clarify your question beforehand. Is the issue you're having the fact that you're unable to access the
notifications
variable which you used previously? If so, what wasnotifications
originally set to/holding before you moved it here?