Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial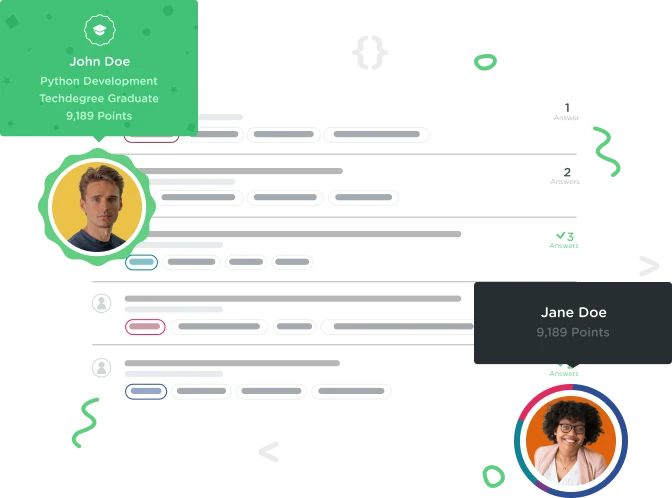

Daniel Toma
2,370 PointsSharing code that prints details for multiple students with the same name
var message = '';
var student;
var answer;
/*
this is the crux of my modifications, to store the student details in an array whenever the name
(from the prompt) is encountered
So, if I enter "Dave" at the prompt, and there are two students named Dave, the details
for each Dave will be pushed (per the array method) onto the message array
Note: this code doesn't account for names not found in the students array
*/
var messageArray = [];
// changed message to messageArray as the incoming argument
function print(messageArray) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = messageArray;
}
function searchForStudent(answer) {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === answer) {
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
/*
Now I populate the messageArray with details of the student when the name matches
but continue because there may be more students with the same name
*/
messageArray.push(message);
/*
It is crucial to now reset the message variable (i.e, details for a particular student)
after pushing the details for a specific student into the array.
Otherwise, the 'message' keeps growing, and each time a new student with the same name
is found, those new details will populate the array as expected, BUT so will the details for
students with the same name that have ALREADY been pushed to the array
*/
message = '';
}
}
return messageArray;
}
answer = prompt("Enter name of student");
while (answer !== "quit") {
messageArray = searchForStudent(answer);
print(messageArray);
answer = prompt("Enter name of student");
}
Steven Parker
231,007 PointsSteven Parker
231,007 PointsWhen you format your code, if you add "js" after the 3 backticks on the top line you'll get nice syntax coloring.