Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial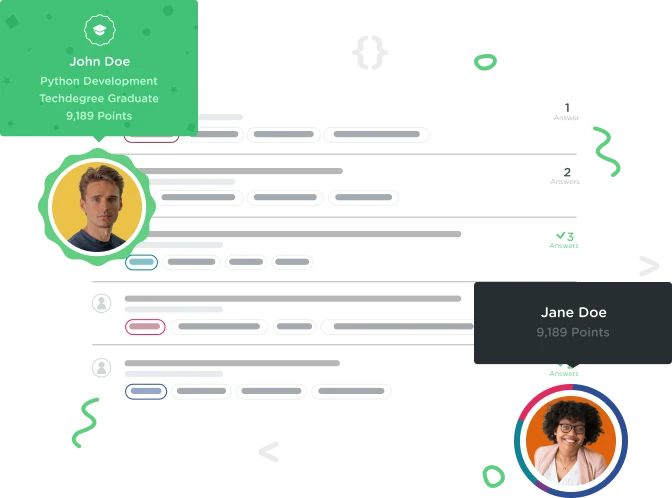
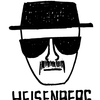
Carlo Sabogal
7,468 PointsSimpler do while loop and why doesnt work.
I don't understand why the program cannot exit the loop on my shorter version of his example. In my code, shouldn't the "guess" variable at some point actually guess the randomNumber and allow the program to exit the loop? When I run this code, I get an infinite loop. Please explain because I don't understand why an exit is needed if at some point guess will be = to randomNumber. thanks!
var upper = 10; var guess; var randomNumber = getRandomNumber(upper);
function getRandomNumber (upperlimit) { return Math.floor(Math.random() * upperlimit)+1; }
do { guess = prompt("guess the number"); } while (guess!==randomNumber)
4 Answers
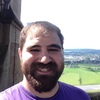
Jamie Perlmutter
10,955 PointsIf you change it to parseInt(guess) !== randomNumber that will fix it. It is because the guess from the prompt is a string and the randomNumber is an integer, once the string from guess is changed to the integer it will compare and once the correct number is put in, it will exit.
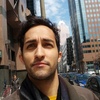
Flavio Carvalho
26,636 PointsI don't really think you can do the:
while( parseInt(guess) !== randomNumber )
The do/while loop need a boolean value to return, either 'true' or 'false', reason why he uses a flag variable in the video. Doing the comparison in the 'while' piece is not really returning the true or false value the loop needs.
Wild guess!
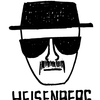
Carlo Sabogal
7,468 PointsOn another video about while loops he does it, which is why this is very confusing.
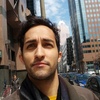
Flavio Carvalho
26,636 PointsOk, maybe I'm going to go a bit too far off, but:
As he explains the do/while loop au contraire from the while loop, tests the condition after it runs the code... so you need the boolean value to be false inside the 'do {}' part of the loop and then the code tests THAT against the 'while()' part of the loop. Did that make sense?
The while loop tests the condition in order to start running the code. The do/while loop runs the code and then tests if it should stop running it, which means you need that boolean value of false before you test against the condition in the 'while()' end of it.
And now my brain just hurt a bit, but logically it sort of makes sense... does it?
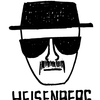
Carlo Sabogal
7,468 PointsFlavio,
I think there was a syntax error somewhere...it works now. Thanks!
var upper = 10;
var randomNumber = getRandomNumber(upper);
var guess;
function getRandomNumber (upper) {
return Math.floor(Math.random() * upper)+1;
}
do{
guess = prompt ('I am thinking of a number between 1 and ' + upper + '. What is it?');
} while(parseInt(guess) !== randomNumber);
document.write (randomNumber);
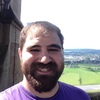
Jamie Perlmutter
10,955 PointsThis worked for me I was able to guess the number and it popped me out.
var upper = 10; var guess; var randomNumber = getRandomNumber(upper);
function getRandomNumber (upperlimit) { return Math.floor(Math.random() * upperlimit)+1; }
do { guess = prompt("guess the number"); } while (parseInt(guess)!==randomNumber)```
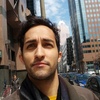
Flavio Carvalho
26,636 PointsWell, my philosophical explanation from before just fell... this worked for me just fine too. So I have no other ideas to what is wrong on Carlo's code!
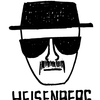
Carlo Sabogal
7,468 Pointsthanks....i had a syntax error somewhere...it works now!
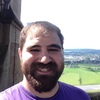
Jamie Perlmutter
10,955 PointsI am glad I could help :-)
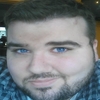
Marcus Parsons
15,719 PointsJust for another perspective, if you wanted users to be able to break out of the program without having to guess the number, you can do this a different way:
var upper = 10;
var guess;
var randomNumber = getRandomNumber(upper);
function getRandomNumber (upper) {
return Math.floor(Math.random() * upper)+1;
}
do{
if (parseInt(guess) !== randomNumber) {
guess = prompt ('I am thinking of a number between 1 and ' + upper + '. What is it?');
}
else {
alert('You guessed the right number! It was ' + randomNumber);
break;
}
//if user just hits enter or hits escape
if (!guess) {
break;
}
} while(true)
Carlo Sabogal
7,468 PointsCarlo Sabogal
7,468 PointsJamie,
I did the change and it still doesn't allow me to exit the loop...my browser crashes.