Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial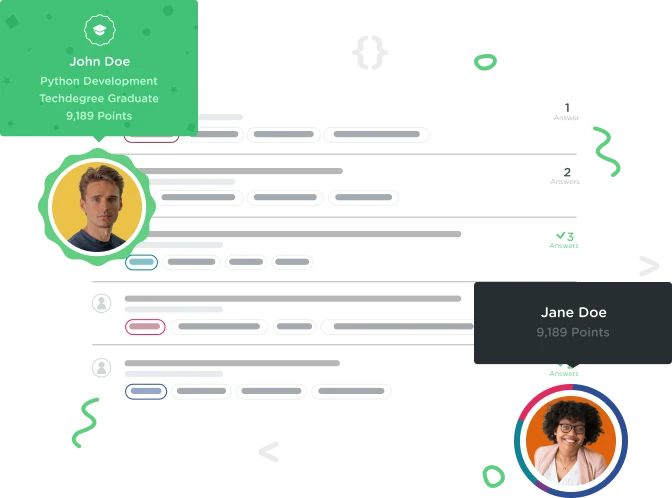

Nijad Kifayeh
6,092 PointsSkipping a property when iterating through an object using a FOR/IN loop
In attempting the Objects code challenge, I came across a small hiccup;
Is there any way to skip a property when iterating through an object using a FOR/IN loop?
Specifically, I want to skip the "name" key.
Here is my codes output:
Student: Nijad
name: Nijad
track: PHP
achv: 1
points: 2
Student: Yumi
name: Yumi
track: JS
achv: 3
points: 4
Student: Nina
name: Nina
track: PHP
achv: 5
points: 6
Student: Mike
name: Mike
track: PHP
achv: 7
points: 8
Student: Chucky
name: Chucky
track: PHP
achv: 9
points: 10
Here is my code:
var students = [
{ name: "Nijad",
track: "PHP",
achv: 1,
points: 2 } ,
{ name: "Yumi",
track: "JS",
achv: 3,
points: 4 } ,
{ name: "Nina",
track: "PHP",
achv: 5,
points: 6 } ,
{ name: "Mike",
track: "PHP",
achv: 7,
points: 8 } ,
{ name: "Chucky",
track: "PHP",
achv: 9,
points: 10 } ,
] ;
var student;
var message = ' ' ;
for (var i = 0; i < students.length; i++ ) {
student = students[i] ;
message += "<h2>Student: " + student.name + "</h2><ul>" ;
/* using a FOR loop to create a single dimension array called student from the students multidimensional array */
for ( var key in student ) {
message += "<li>" + key + ": " + student[key] + "</li>" ;
}
/* using a FOR/IN loop to iterate through each property of the student array */
message += "</ul>" ;
}
function print( message ) {
var outputDiv = document.getElementById( 'output' ) ;
outputDiv.innerHTML = message ;
}
print( message ) ;
1 Answer
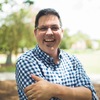
Dave McFarland
Treehouse TeacherYes! Just use a conditional statement inside the for...in
loop to check for the key name:
for ( var key in student ) {
if (key !== 'name') {
message += "<li>" + key + ": " + student[key] + "</li>" ;
}
}
Nijad Kifayeh
6,092 PointsNijad Kifayeh
6,092 Pointsah..so simple! Thanks Dave!!
Nijad Kifayeh
6,092 PointsNijad Kifayeh
6,092 PointsHaving said that....I can't figure out what's wrong with the code now, can you take a look please?
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherYou don't have the
print()
function in this code, and you don't ever call a function to print to the page.