Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial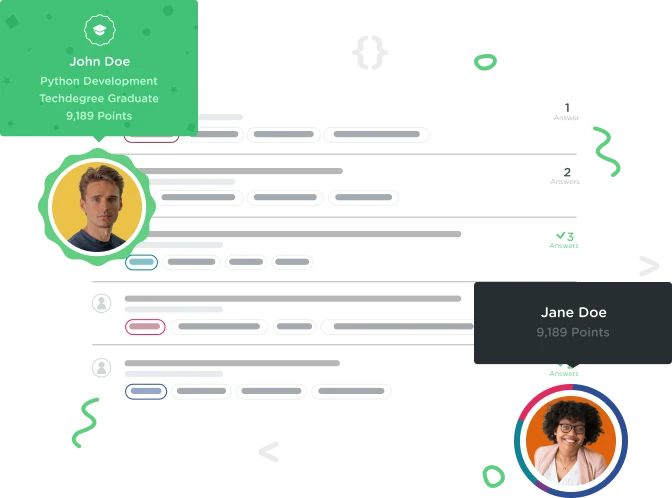

Hugo Jeffreys
Python Web Development Techdegree Student 1,738 PointsSlice challenge 4 of 4, code not working in Treehouse, but fine in idle editor
Not quite sure why this isn't working out for me within the challenge as it seems fine when put to the test in IDLE. Many thanks!!
def first_4(word):
return word[:4]
def first_and_last_4(word):
return word[:4] + word[-4:]
def odds(word):
return word[1::2]
def reverse_evens(word):
return word[-1::-2]
2 Answers
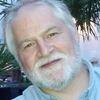
Jeff Muday
Treehouse Moderator 28,720 PointsYes... you are very close. There are some unit test cases on which your method will not pass-- e.g. lists with an even number of elements. This is very subtle, but the challenge wants you to generate evens and then reverse, rather than reverse the list, and generate the evens.
There are a bunch of ways to do this correctly, though this concise methodology will work:
- first, generate the even elements of the list; word[::2]
- next, reverse the list; word[::-1]
combine these... and you will have the answer you were going for. word[::2][::-1]
Python 3.6.4 (v3.6.4:d48eceb, Dec 19 2017, 06:04:45) [MSC v.1900 32 bit (Intel)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> # first here is some test data
>>> x = [1,2,3,4,5]
>>> y = [1,2,3,4,5,6]
>>> # here is a list reversal--
>>> x[::-1]
[5, 4, 3, 2, 1]
>>> # here is your function below
>>> def reverse_evens(word):
... return word[-1::-2]
...
>>> # now test it on list x and it will work properly, len of 5
>>> reverse_evens(x)
[5, 3, 1]
>>> # but this will produce incorrect results on y
>>> reverse_evens(y)
[6, 4, 2]
>>> # now try the suggested solution, notice the results differ on list y which has len of 6
>>> x[::2][::-1]
[5, 3, 1]
>>> y[::2][::-1]
[5, 3, 1]
>>>

Hugo Jeffreys
Python Web Development Techdegree Student 1,738 PointsThanks Jeff. It took me a while to realise the devil was in the detail (i.e. the question was asking for even numbers, not just count backwards in pairs), but I got there in the end by using using an 'if' loop. Having seen your example though, your code seems much more elegant. I hadn't picked up that you could just tag on another index on the end of the previous one like that. Many thanks for your time and teaching!
Michael Russell
Courses Plus Student 8,550 PointsMichael Russell
Courses Plus Student 8,550 PointsThis was an excellent answer, thank you.