Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial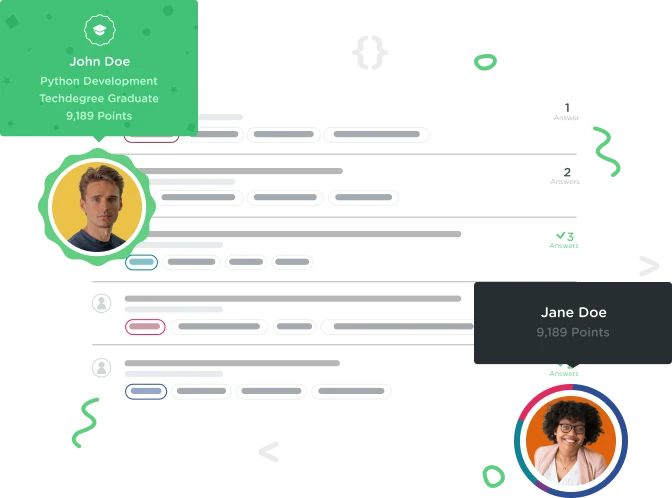

Mikaela Land
Python Web Development Techdegree Student 2,324 PointsSlice Functions: Local variable error
In the odds function I keep getting:
UnboundLocalError: local variable 'odds_only' referenced before assignment
Not sure how to fix it. I keep trying to change things around to test it but I've got nothing. I got it to work once but it stopped working the second time.
Any ideas?
def first_4(lst):
return lst[:4]
def first_and_last_4 (lst2):
first = lst2 [:4]
last = lst2 [-4:]
both = first + last
return both
def odds (num):
if len(num) % 2 == 0:
odds_only = num [1::2]
return odds_only
1 Answer
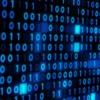
Alexander Davison
65,469 PointsI think you are a bit lost. The odds
function takes in an iterable still, not a number. (It seems that you believe that it takes in an iterable.)
The problem is asking to return an iterable which has all of the odd indexed values from the passed in iterable.
For instance, if I pretended I was within the Python shell:
>>> odds([1, 2, 3, 4, 5])
[2, 4]
>>> odds('abcdefg')
['b', 'd', 'f']
>>> odds((1, 2, 3))
[2]
Here's my solution to this problem:
(Let's call the input iterable iter
and the output iterable result_iter
)
- Go through every number from zero to N-1, where N is
len(iter)
. Let's call the "current number"i
. - If
i
is odd, add toresult_iter
iter[i]
. (Becausei
is an index, not the item itself) - Return
result_iter
.
Implemented in code, it may look somewhat like this:
def odds(iter):
result_iter = []
for i in range(len(iter)):
if i % 2 != 0:
result_iter.append(iter[i])
return result_iter
Simpler way for the Pythonista:
def odds(iter):
return [iter[i] for i in range(len(iter)) if i % 2 != 0]
I hope this helps!
Happy coding! ~Alex
Mikaela Land
Python Web Development Techdegree Student 2,324 PointsMikaela Land
Python Web Development Techdegree Student 2,324 PointsThanks. I think I get it. I just have a question: why did you add range? Wouldn't that imply the list is just numbers? What's its purpose in the code?