Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial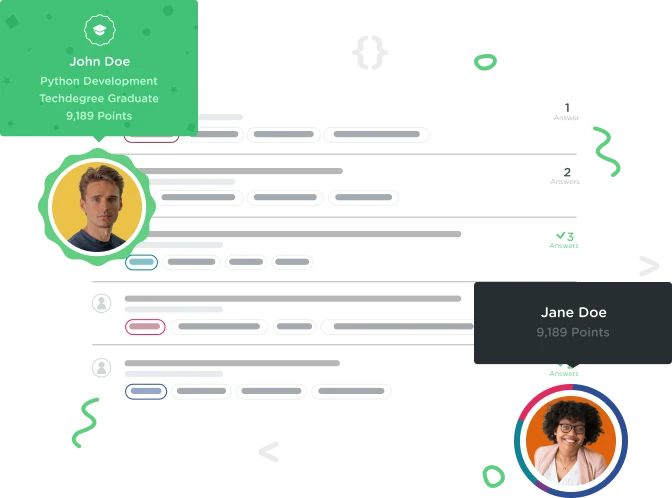
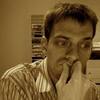
Joseph Puchalik
Courses Plus Student 4,029 PointsSlight differences in JS from the "official" answer... can someone review and explain if mine is flawed and why?
This is JS I came up with on my own (which I was quite happy with). It SEEMS to work. But I haven't had time to enter a couple million different numbers into it to see if it generates a number outside the range...
var rangeFloor = parseInt( prompt('Enter the low number in your range:') );
var rangeCeiling = parseInt( prompt('Enter the high number in your range:') );
var numGen = Math.ceil( Math.random() * (rangeCeiling - rangeFloor) ) + rangeFloor;
document.write('Your random number is: ' + numGen);
2 Answers
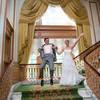
Colin Bell
29,679 PointsVery solid. However, you're going to want to use Math.floor instead of Math.ceil() in your numGen variable. While Math.random() can technically generate a 0, the actual chances of that happening are extraordinarily slim.
So your random number generation is for all intents and purposes generating a number between 1 to rangeCeiling
instead of 0 to rangeCeiling
and then adding that to your rangeFloor
. If you're trying to find a random number within a large range you'll probably never notice, but it becomes easily noticeable in small ranges. For example, if you're trying to find a random number between 1 and 2 would always be 2, a random number between 4 and 5 would always be 5, etc.
var rangeFloor = 4;
var rangeCeiling = 5;
Math.ceil ( Math.random() * (rangeCeiling - rangeFloor) ) + rangeFloor;
// Math.ceil ( Math.random() * (5 - 4) ) + 4;
// Doesn't matter if Math.random() * ( 5 - 4 ) is equal to .00001 or .999999,
// Math.ceil() will always push that up to 1
// So that plus the rangeFloor will always === 5 in this case.
Math.random() will never return 1, so getting the Math.floor on the range plus 1 then adding the rangeFloor
to that will find a true random number between your rangeFloor
and your rangeCeiling
var rangeFloor = 1;
var rangeCeiling = 2;
Math.floor ( Math.random() * (rangeCeiling - rangeFloor + 1) ) + rangeFloor;
So if Math.random() generates 0.49 then 0.49 * ( 2 - 1 + 1)) === 0.98. The floor of that is 0. Add 1 and you get 1.
if Math.random() generates 0.99 then 0.5 * ( 2 - 1 + 1)) === 1.98. The floor of that is 1. Add 1 and you get 2.
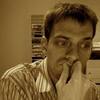
Joseph Puchalik
Courses Plus Student 4,029 PointsOK, thank you for your help. I had considered small ranges but I never tested or considered ranges where the rangeFloor and rangeCeiling were consecutive numbers.
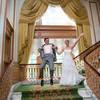
Colin Bell
29,679 PointsI just used consecutive numbers to easily show it. If you were to use 4 as the rangeFloor and 6 as the rangeCeiling you'd only get 5 or 6, never 4. With a rangeFloor and rangeCeiling 2 and 7, you'd only ever get 3, 4, 5, 6, or 7. With Math.ceil() you'd (almost) never get the number you put in as the rangeFloor - unless Math.random() returned its mythical 0.