Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial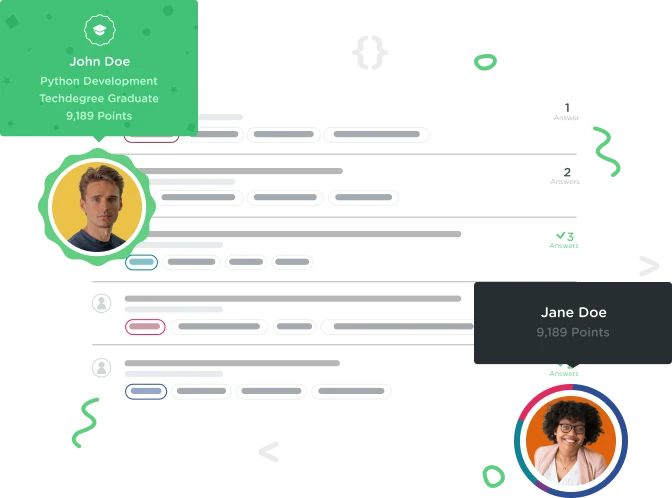

Susan Rusie
10,671 PointsSo close to a solution but not sure what I am doing wrong
I am really having trouble with this bonus challenge. It will show that student doesn't exist, but it does it for all of them.. If I type in a legitimate name, it might not show it, but hitting cancel on the prompt will. I also am not sure what to do to get it to display the information of students with the same name. Any help on this would be greatly appreciated.
Here is my code:
var message = '';
var student;
var search;
var existence;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport ( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name {Jody] (or type "quit" to end');
if (search === null || search.toLowerCase() === 'quit' ) {
break;
} else {
message = (prompt)("<p>That student doesn't exist. Please try again</p>");
print(message);
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message = getStudentReport ( student );
print(message);
}
}
}
2 Answers
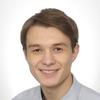
Sergey Podgornyy
20,660 PointsWorking code as an example. Comments to it you can watch below.
var message;
var students = [
{
name: 'Jody',
track: 'math',
points: 99,
achievements: 'best student ever!'
},
{
name: 'Sally',
track: 'math',
points: 10,
achievements: 'worst student ever!'
},
{
name: 'Kim',
track: 'math',
points: 100,
achievements: 'best of the best student ever!'
}
];
var searchingName;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport ( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
function searchForStudent (searchingName) {
for (var i = 0; i < students.length; i += 1) {
if ( students[i].name === searchingName ) {
return students[i];
}
}
return false;
}
while (true) {
searchingName = prompt('Search student records: type a name {Jody} (or type "quit" to end)');
if (searchingName === "" || searchingName.toLowerCase() === 'quit' || searchingName === null) {
message = "<p>Try to Enter something!</p>";
print(message);
break;
} else {
if (searchForStudent (searchingName)) {
message = getStudentReport ( searchForStudent (searchingName) );
print(message);
break;
} else {
message = "<p>That student doesn't exist. Please try again</p>";
print(message);
break;
}
}
}
First of all you wont see result, because each time you will see an infinite prompt that asks you to enter something(as was said before). Second thing. When I'm typing something wrong(wrong name for example), it will eventually output error message and then go to the for loop and continue to search for name that doesn't exist, and after that search it will prompt to enter something again. So, the idea is to search first and then output the result ;)
In code above first thing that you need to check if user enter empty value, or 'quit', or press cancel button. If he did that, then you can stop iterating and go out of the loop. If he actually enter something, then you need to check if this something exist at all :) For this purpose you can use searchForStudent() function which returns student if the name is correct, and false if he doesn't.
After checking that in else statement you can decide what output will look like. And most important to see that output. Hope, that this post explained how things work. ;)
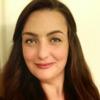
Jennifer Nordell
Treehouse TeacherIt's sort of hard to read your code without the markdown, but I can point out one thing I think might be your culprit. Take a look at this part:
if (search === null || search.toLowerCase() === 'quit' )
{
break;
} else {
message = (prompt)("<p>That student doesn't exist. Please try again</p>");
print(message);
}
Here you're saying if your search is null or quit then break. But if it wasn't null then tell the user that the student doesn't exist. So it seems like unless the search is null or quit it's going to tell you that student doesn't exist... even if they do.