Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial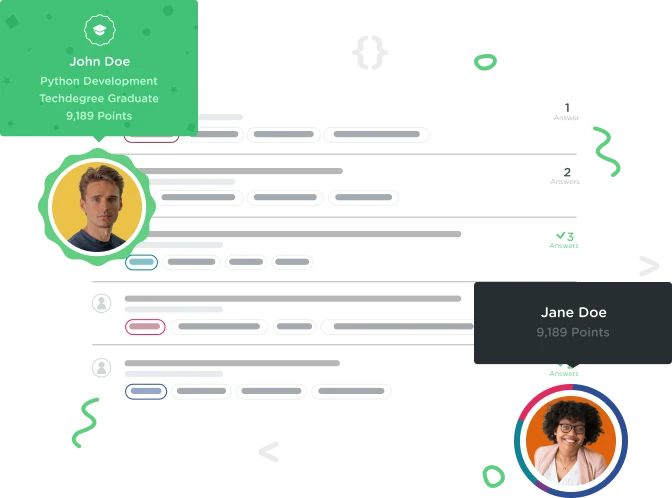
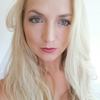
Lotte Bloem
18,630 Pointsso far my solution, but is there a way that it doesn't matter if i write the name in capital or not..
var message = '';
var student;
var search;
var students = [
{name: 'Lotte', track: 'JavaScript', achievements: '58', points: '1730'},
{name: 'Damien', track: 'iOS', achievements: '175', points: '16375'},
{name: 'Sandra', track: 'PHP Development', achievements: '155', points: '15025'},
{name: 'Marc', track: 'Phyton', achievements: '40', points: '1950'},
{name: 'Laureen', track: 'Css', achievements: '5', points: '350'}
];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while(true) {
search = prompt('Search the student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
} else {
print ( 'No student named ' + search + ' was found');
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search ){
message = getStudentReport( student );
print(message);
}
}
}
3 Answers

Dustin Matlock
33,856 PointsHi Lotte, JavaScript is case-sensitive.

Yonay Gonzalez
8,064 PointsHello Lotte,
Here is my solution. It prints only the records with same name and prints a message if there is no one with that name.
I hope it helps you.
var message = '';
var student;
var search;
var results = [];
var studentReport;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudents(student) {
studentReport = "<h2>Student: " + student.name + "</h2>";
studentReport += "<p>Track: " + student.track + "</p>";
studentReport += "<p>Achievements: " + student.achievements + "</p>";
studentReport += "<p>Points: " + student.points + "</p>";
return studentReport;
}
while (true) {
search = prompt("Please type a name or type 'quit' to exit.");
if (search === null || search.toLowerCase() === "quit") {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
results.push(getStudents(student));
message = results.join("");
}
if (results.join("") === "") {
message = "<br><h2>Sorry, no " + search.toUpperCase() + " listed here.</h2>";
}
}
print(message);
results = [];
}
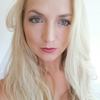
Lotte Bloem
18,630 PointsThanks Yonay for helping me!
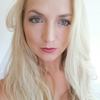
Lotte Bloem
18,630 Pointsthanks! And i had another question what if i have 2 students with the same name, how can i make the code right that i can find both students, and not only the last one?

OMER BALAK
4,255 PointsJust change message= to message += so duplicate names will display ! while(true) { studentName = prompt("Whats the name of the student?"); if (studentName.toLowerCase() === 'quit' || studentName === null) { break; }
for (var i = 0; i < students.length; i += 1) { student = students[i]; if (studentName.toLowerCase() === student.name.toLowerCase()) { message += getStudentReport(student); print(message); }
}
}