Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial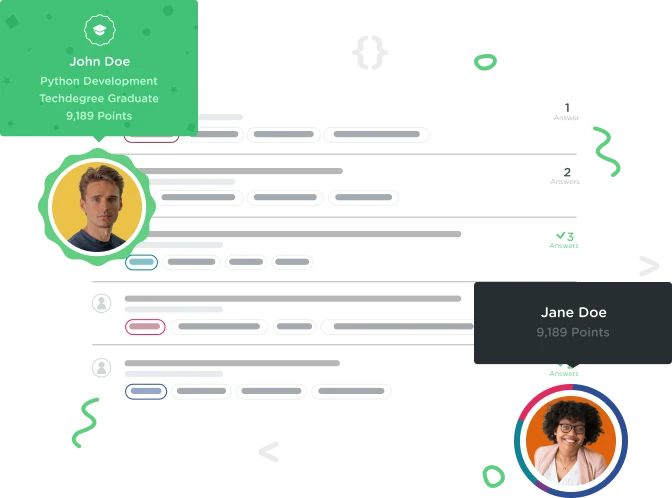
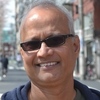
GURUPRASAD NAGARAJAN
7,853 Pointssolution?
code works but list shows empty:
def show_help(): print("What to pick up at store?") print(""" Enter'DONE' to stop adding items Enter HELP for help menu Enter SHOW to view list """) def show_list(shopping_list): print("Heres the list:") for item in shopping_list: print(item)
def add_to_list(shopping_list, new_item): shopping_list = [] shopping_list.append(new_item) print("Added {}. List has {} items".format(new_item, len(shopping_list))) return shopping_list
def main(): while True: show_help() shopping_list = [] new_item = input("> ") # //in python 3 version it's input("> ") if new_item == 'DONE': break elif new_item == 'HELP': show_help() continue elif new_item == 'SHOW': show_list(shopping_list) continue add_to_list(shopping_list,new_item) show_list(shopping_list) main()
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
def main():
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
main()
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
4 Answers
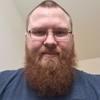
Henrik Christensen
Python Web Development Techdegree Student 38,322 Pointsdef show_help():
print("What to pick up at store?")
print("""
Enter'DONE' to stop adding items
Enter HELP for help menu
Enter SHOW to view list
""")
def show_list():
print("Heres the list:")
# this function don't know what shopping_list is
# try pass shopping_list as a parameter to this function
# def show_list(shopping_list)
for item in shopping_list:
print(item)
def add_to_list(new_item):
# same problem here
# function does not know what shopping_list is
shopping_list.append(new_item)
print("Added {}. List has {} items".format(new_item, len(shopping_list)))
def main():
shopping_list = []
show_help()
while True:
new_item = input("> ")
# //in python 3 version it's input("> ")
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list() # remember to update code here if you add parameter to the function
continue
add_to_list(new_item) # remember to update here too
# since show_list don't know what shopping_list is
# then you should not be getting output from show_list() here
# remember to update if you add parameter to the function
show_list()
main()
I hope the comments is helpful :-)
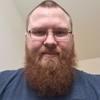
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsI see 2 problems:
- You're calling main() inside your while-loop which is going to create an empty list for each time inside the loop
while True:
main() # remove this line
- You have not indented your if / elif / else properly - they are outside the while-loop
while True:
main()
# ask for new items
# code below this comment is not inside the while-loop
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
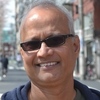
GURUPRASAD NAGARAJAN
7,853 PointsHi Henrik, Thanks a lot for your quick response, appreciate it. I re-did the code, it works but when I do DONE, it throws 'NameError: name 'shopping_list' is not defined: def show_help(): # print out instructions on how to use the app print("What should we pick up at the store?") print(""" Enter 'DONE' to stop adding items. Enter 'HELP' for this help. Enter 'SHOW' to see your current list. """)
def show_list(shopping_list): # print out the list print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item): # add new items to our list shopping_list.append(new_item) print("Added {}. List now has {} items.".format(new_item, len(shopping_list))) return shopping_list
def main(): show_help() # make a list to hold onto our items shopping_list = [] while True: # ask for new items new_item = input("> ") # be able to quit the app if new_item == 'DONE': break elif new_item == 'HELP': show_help() continue elif new_item == 'SHOW': show_list(shopping_list) continue add_to_list(shopping_list, new_item)
main() show_list(shopping_list)
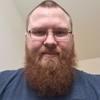
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsAny chance you could format that code? because it's impossible to see indentations etc. when it's printed like that
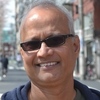
GURUPRASAD NAGARAJAN
7,853 Pointsshopping_list = [] Hi Henrik, Sorry for the delay I was trying to play around and see if I can do it, things the below code works on my mac and the workspace (I've tried to do markdown by ```python before the code, hope that displays properly) but if I don't put shopping_list = [] in the beginning, I get 'shopping_list not defined' error. Thanks a lot for your help.
def show_help():
print("What to pick up at store?")
print("""
Enter'DONE' to stop adding items
Enter HELP for help menu
Enter SHOW to view list
""")
def show_list():
print("Heres the list:")
for item in shopping_list:
print(item)
def add_to_list(new_item):
shopping_list.append(new_item)
print("Added {}. List has {} items".format(new_item, len(shopping_list)))
def main():
shopping_list = []
show_help()
while True:
new_item = input("> ")
# //in python 3 version it's input("> ")
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list()
continue
add_to_list(new_item)
show_list()
main()
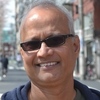
GURUPRASAD NAGARAJAN
7,853 PointsHi Henrik, Thanks so much for your patience and help. I must have pasted the wrong code as I already had shopping_list as param in the previous attempt. But took your advice and made necessary changes, the code works on my mac and n the workspace. But fails the test somehow, so I am not going to bother with it anymore as I know the code works. Here's the code (it also works without calling the show_list(shopping_list) in the end):
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def show_list(shopping_list):
print("Here's your list:")
# print out the list
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
def main():
shopping_list =[]
show_help()
# make a list to hold onto our items
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
main()