Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial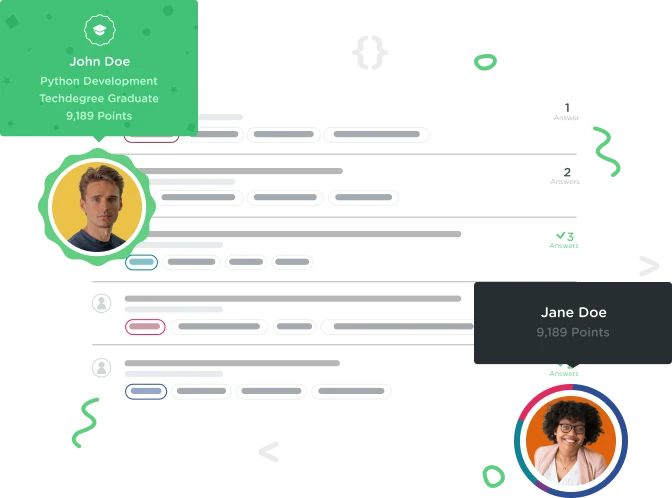

Hermann Azong
3,176 Pointssolution
var html = ''; var red; var green; var blue; var rgbColor;
function generateRGB(color){ color = Math.floor(Math.random() * 256 ); return color; }
for( var i = 0; i < 10; i += 1){ rgbColor = 'rgb(' + generateRGB(red) + ',' + generateRGB(green) + ',' + generateRGB(blue) + ')'; html += '<div style="background-color:' + rgbColor + '"></div>'; }
document.write(html);
5 Answers

vaetev
Java Web Development Techdegree Student 11,026 Pointsvar html = '';
for(var i = 0 ; i < 100 ; i++){
function rand(){
return Math.floor(Math.random() * 256);
}
html += '<div style="background-color:rgb(' + rand() + ',' + rand() + ',' + rand() + ')"></div>';
}
document.write(html);
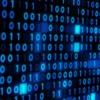
Alexander Davison
65,469 Points Looks great!

Hermann Azong
3,176 Pointsthx Alexander!

Ryan Moody
20,499 Pointsvar html = ''; var red; var green; var blue; var rgbColor;
for (var i = 1; i <= 10; i =+ 1){ red = Math.floor(Math.random() * 256 ); green = Math.floor(Math.random() * 256 ); blue = Math.floor(Math.random() * 256 ); rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')'; html += '<div style="background-color:' + rgbColor + '"></div>'; }
document.write(html);
This was my code, but i am getting an "allocation size overflow". The code is the same as the instructors.

Edwin Carbajal
4,133 PointsHi Ryan,
Not sure if you have found a solution to your problem but let me show you anyway. It looks like you have a typo in your for loop. Here:
for (var i = 1; i <= 10; i =+ 1)
The i variable should increase each loop. And the correct syntax for this is:
i += 1

Marina Shumeyko
5,647 Pointsbetter to write for (var i = 1; i <= 10; i++)
i++ // that is more common practice
is the same as
i += 1
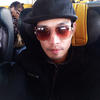
rayjens code
882 Pointsw0w, i wish my brain is like yours Alexandra and Vaetev :) I solve mine like this so poor logic >.<
"var html = ''; var red; var green; var blue; var rgbColor; var count=0;
while (count<100){
red = Math.floor(Math.random() * 256 ); green = Math.floor(Math.random() * 256 ); blue = Math.floor(Math.random() * 256 ); rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')'; html += '<div style="background-color:' + rgbColor + '"></div>'; count+=1; }"
Alexandra Wakefield
1,866 PointsAlexandra Wakefield
1,866 PointsIt seems I did the same. The only difference is I omitted the variables red, blue, green and just when with repeating the function name.