Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial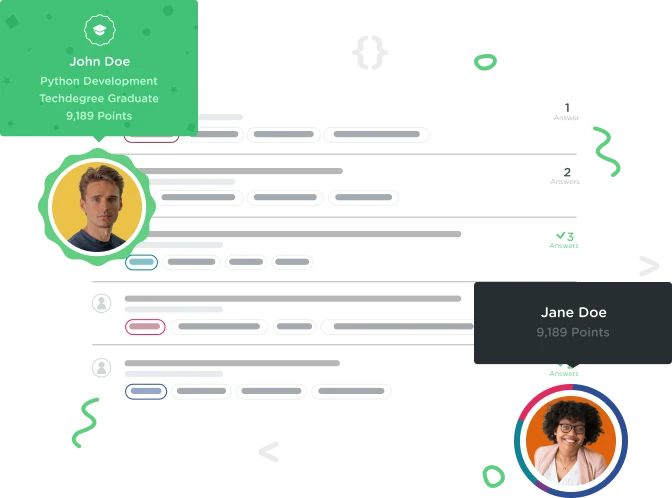
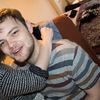
Marc Murray
4,618 PointsSolution broke after attempting to optimize the code.
var questions = [
['What year was Marc born?', '1992'],
['What day was Marc born?', '9'],
['What month was Marc born?', '2']
];
var rightAnswers = 0;
var wrongAnswerCount = 0;
var wrongAnswerList = [];
var rightAnswerList = [];
var listHTML;
function buildList(arr) {
var listHTML = '<ul>';
for (var i = 0; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ul>';
return listHTML;
}
function ask() {
for (var questionCount = 0; questionCount <= (questions.length - 1); questionCount += 1) {
var answer = prompt(questions[questionCount][0]);
console.log(questionCount);
if (answer === questions[questionCount][1]) {
rightAnswers += 1;
rightAnswerList.push(questions[questionCount][0]);
} else {
wrongAnswerCount += 1;
wrongAnswerList.push(questions[questionCount][0]);
}
}
document.write('<p> Great! You got ' + rightAnswers + ' correct!' + '</p>');
document.write('<p> Here are the ones you got right:</p>');
buildList(rightAnswerList);
document.write(listHTML);
if (wrongAnswerCount > 0) {
document.write('<p>You got ' + wrongAnswerCount + ' wrong too, these ones:');
buildList(wrongAnswerList);
document.write(listHTML);
}
}
ask();
I had this working earlier, before I tried to mimic the buildList function, since adding that in I am getting "undefined" rather than the lists being built. Can anyone see what I'm missing?
1 Answer

Philip G
14,600 PointsHi Mark,
the function buildList()
doesn't return listHTML
as global variable, it returns just the value at calling the function. listHTML
is only valid within the function
This Code should work:
var questions = [
['What year was Marc born?', '1992'],
['What day was Marc born?', '9'],
['What month was Marc born?', '2']
];
var rightAnswers = 0;
var wrongAnswerCount = 0;
var wrongAnswerList = [];
var rightAnswerList = [];
var listHTML;
function buildList(arr) {
var listHTML = '<ul>';
for (var i = 0; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ul>';
return listHTML;
}
function ask() {
for (var questionCount = 0; questionCount <= (questions.length - 1); questionCount += 1) {
var answer = prompt(questions[questionCount][0]);
console.log(questionCount);
if (answer === questions[questionCount][1]) {
rightAnswers += 1;
rightAnswerList.push(questions[questionCount][0]);
} else {
wrongAnswerCount += 1;
wrongAnswerList.push(questions[questionCount][0]);
}
}
document.write('<p> Great! You got ' + rightAnswers + ' correct!' + '</p>');
document.write('<p> Here are the ones you got right:</p>');
document.write(buildList(rightAnswerList));
if (wrongAnswerCount > 0) {
document.write('<p>You got ' + wrongAnswerCount + ' wrong too, these ones:');
document.write(buildList(wrongAnswerList));
}
}
ask();
Referrence: https://developer.mozilla.org/de/docs/Web/JavaScript/Reference/Statements/return
Best Regards,
Philip