Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial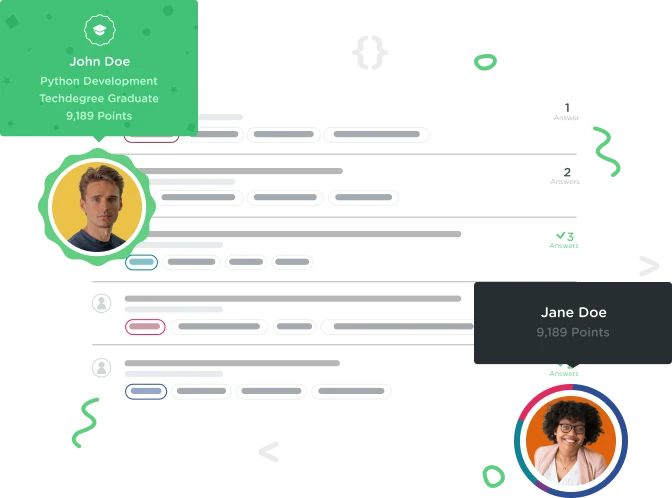
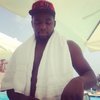
Edwin Billa
Courses Plus Student 6,882 PointsSolution for Extra Credit! Student Record Search Challenge. Please Review my code and let me know. Thanks
// Array of students information
// i duplicated some names so we can check outcome
// if that name is provided as input!
var students = [
{
name: 'dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'john',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'trish',
track: 'Rails Development',
achievements: '5',
points: '350'
},
{
name: 'allen',
track: 'Learn Ruby',
achievements: '45',
points: '2300'
},
{
name: 'dave',
track: 'Android Development',
achievements: '15',
points: '350'
}
];
And below is the main.
//function to write the info on the document.
function print(message) {
var outPutDiv = document.getElementById('output');
outPutDiv.innerHTML = message;
}
var studentLog;
var html ="";
var search;
var noInfo = "";
//function to capitalize first Letter of a string
function firstLetterCapitalize(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
// function that returns the student info into the variable html
function studentInfo() {
html += "<h2>Student: " + firstLetterCapitalize(studentLog.name) + "</h2>";
html += "<p>Track: " + studentLog.track + "</p>";
html += "<p> Points: " + studentLog.points + "</p>";
html += "<p> Achievements: " + studentLog.achievements + "</p>"
return html;
}
// function that returns a message when student name is not found!
//it returns the message into the variable noInfo
function notFound() {
noInfo += "<h2>No Info found for '";
noInfo += firstLetterCapitalize(search);
noInfo += "'. Please search again!</h2>";
return noInfo;
}
// a while loop to repeat the whole process infinetly untill
//the conditional with the break statement is true
while(true) {
search = prompt("Please search by entering a name 'eg. Jody' Press cancel button or type 'quit' to end the program!");
if(search === null || search.toLowerCase() === 'quit'){
break;
}
// iterating throught the students array
for (var i = 0; i < students.length; i++) {
studentLog = students[i];
//checking if the input matches any name
//in the any of the object in the array
//if it does then run the studentInfo() function
if(search.toLowerCase() === studentLog.name) {
studentInfo();
}
}
//out of the For loop to check if the input doesn't
//match any name in the any of the object in the array
//if it doesn't run the notFound() function
if (search.toLowerCase() !== studentLog.name) {
notFound();
}
// conditional to check if the html variable is empty or not!
//if not empty print the html value. if it's empty then that means
//no correct match...print the noInfo value
if (html.length > 0) {
print(html);
}
else {
print(noInfo);
}
//re-initialize the html and noInfo variables to an empty screen
//to avoid print the previous info each time the loop run
noInfo="";
html = "";
}
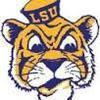
Cena Mayo
55,236 PointsLooks awesome, Edwin! I particularly appreciate your code comments. As someone who gets easily confused :D they're a great way to break down your code and see what's happening at each stage. Thanks for posting this!
6 Answers

Chetan Jaisinghani
2,896 PointsHave you checked you console for any errors?
What I don't understand is:
if studentsLog = students[i];
How is this even executing outside the for loop:
if (search.toLowerCase() != studentLog.name)
This statement clearly requires the value for i
So how can the values for i iterate outside the for loop?
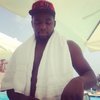
Edwin Billa
Courses Plus Student 6,882 PointsHey Chetan, to correct your concerns...i think you should look at the code again. you probably didn't see well. All the statements are executing inside the for loop... they ending curly bracket might have escaped you. i hope it helps :)

Chetan Jaisinghani
2,896 Points//out of the For loop to check if the input doesn't //match any name in the any of the object in the array //if it doesn't run the notFound() function if (search.toLowerCase() !== studentLog.name) { notFound(); }
It clearly says 'out of the for loop'
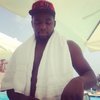
Edwin Billa
Courses Plus Student 6,882 PointsOh i see now what you meant. The code executes out of the loop because I declared the studentLog variable as a global variable. so it can be accessed anywhere in the program. i only initialised the studentLog variable to student[i] from the loop so its value is still accessible outside the loop.

Kymchy Slice
3,825 PointsLovely solution, I couldnt get multiple names to print :)
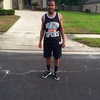
Darius Stewart
2,543 PointsHey, is the 'firstLetterCapitalize' function needed, also what is it's purpose?
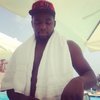
Edwin Billa
Courses Plus Student 6,882 PointsI incorporated that just for semantics. It just output the changes the first letter of the name to Capital incase it was entered as lower case. Names are suppose to be upper case at all times
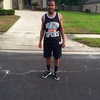
Darius Stewart
2,543 PointsIt def does not work if you take the firstLetterCapitalize function out, I'm confused as to why
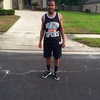
Darius Stewart
2,543 PointsI also notice that it does not work when you capitalize the name values in the students array, can you please explain that if you don't mind
Edwin Billa
Courses Plus Student 6,882 PointsEdwin Billa
Courses Plus Student 6,882 PointsI think this does everything mentioned in the challenge with extra credit :)