Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial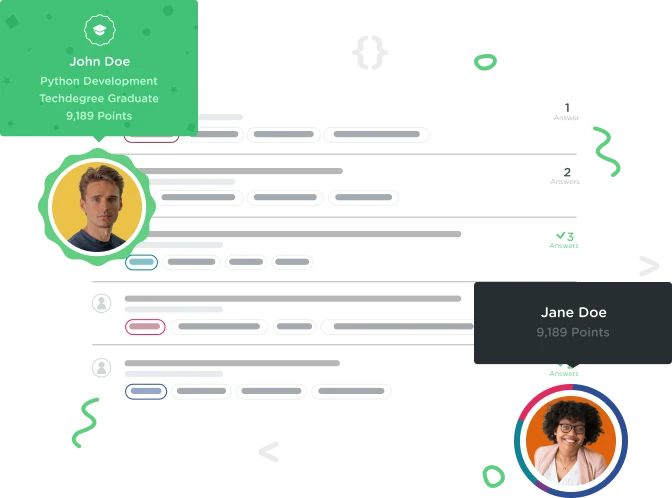

Anthony Olugbusi
15,585 PointsSolution review, any feedback greatly appreciated
//Questions
var quiz=[['How many states make up the US?','50'],
['How many continets are there in the world?','7'],
['How many days make up a year?','365 days' ]];
//Answer store
var correctAnswer=[];
var incorrectAnswer=[];
//function which identifies correct and incorrect answers, also updates 'Answer store' lists
function quizSorter(arrlist){
for(var i=0; i<arrlist.length;i+=1){
var question=arrlist[i][0];
var answer=arrlist[i][1];
var response=prompt(question);
if(response===answer){
correctAnswer.push(question)
}
else{
incorrectAnswer.push(question)
}
}
}
//function which creates an ordered HTML list
function listMaker(arrlist){
var listHTML='<ol>';
for(i=0;i<arrlist.length;i+=1){
listHTML+='<li>'+arrlist[i]+'</li>';
}
listHTML+='</ol>';
return listHTML
}
//default print function
function print(message) {
document.write(message);
}
quizSorter(quiz);
html = "You got " + correctAnswer.length + " question(s) right out of "+quiz.length
html +='<h2>You got these questions correct:<h/2>';
html +=listMaker(correctAnswer);
html +='<h2>You got these questions incorrect:<h/2>';
html +=listMaker(incorrectAnswer);
print(html)
I primarily come from a C/C++/C# and python background, so any feedback on what I could have done to reduce any unnecessary long code is greatly appreciated.
I am trying to go for very simplistic approach.
1 Answer

Thomas Nilsen
14,957 PointsNothing wrong here really. Here is my main suggestions though:
- Add spaces. It becomes so much more readable.
- you store questions and answers in an 2D array. An object might be more suitable.
- When the if / else is very straight forward, ternary operators saves you a couple of lines of code.
Here is your rewritten code, with the suggested improvements.
var quiz = [
{question: 'How many states make up the US?', answer: '50'},
{question: 'How many continets are there in the world?', answer: '7'},
{question: 'How many days make up a year?', answer: '365 days'}
];
var correctAnswer = [];
var incorrectAnswer = [];
function quizSorter(arr) {
arr.forEach(function(obj) {
var response = prompt(obj.question);
(response === obj.answer ? correctAnswer : incorrectAnswer).push(obj.question);
});
}
quizSorter(quiz);