Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial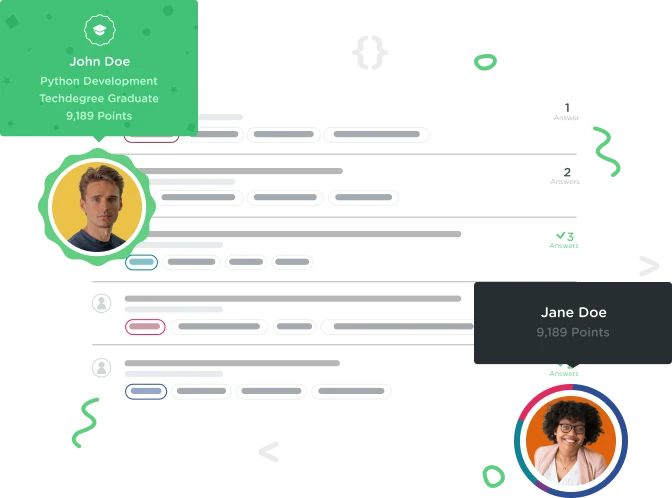

Gustavo Florez
14,811 PointsSolution to both features: - Displaying a message when student is not found. - Displaying all students with same name.
I hope this helps anyone trying to solve the extra challenges.
- When a user gives a student name that is not in the list we can keep track of this by adding a counter in the "else" condition of our code. This counter holds the number of all the students the search value has been compared to, which is equal to the length of the array. This means that when our counter is equal to the length of the array of students the user has given a name which is not in the list. We can then display a message to the user when this condition is met, telling the user the student is not found. After this we reset the counter so that the next search can be compared again.
- To display all students with the same name we use the message variable and construct our string inside this variable calling the function getStudentReport(). It is important to note that if not using += or message = message + getStudentReport() but instead just suing message = getStudentReport(), you will only be able to print one student! So pay attention to this small detail which caused me a few hours of work...
var message = '';
var student;
var search;
var notFound = 0;
function print() {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Search student records: type a name [Jody] (or type 'quit' to end)");
search = search.toLowerCase();
if (search === null || search === 'quit') {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search) {
message += getStudentReport(student);
} else {
notFound += 1;
}
}
if (notFound === students.length) {
alert('Student not found, please try again or type "quit" to exit.');
notFound = 0;
} else {
alert('Student found! Type "quit" to display your results.');
}
print(message);
}
4 Answers
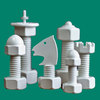
Steven Parker
230,995 PointsAnother way to determine if a student was not in the list is to set a boolean variable before the loop, and then toggle the value when a match is found.
An advantage of this method is that no "else" is needed in the matching test.
var found = false; // preset success flag (to false)
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search) {
message += getStudentReport(student);
found = true; // set success flag
} // no "else" needed
}
if (!found) { // was nothing found?
// other code the same ...

ICHEN WU
Courses Plus Student 5,427 Pointsnice work! thanks for sharing!

JORGE HERRANDO GARIJO
7,895 PointsThat's true. I thought it was at the beginning of the code. My apologies.
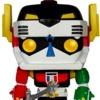
alee2
5,435 PointsThank you for sharing this!

Gustavo Florez
14,811 PointsYour welcome :)
JORGE HERRANDO GARIJO
7,895 PointsJORGE HERRANDO GARIJO
7,895 PointsHi there!
Great solution above. Congrats!
I would just like to add that once you set found to 'true', it remains 'true' while the 'while' loop is running, even if the next 'search' does not match any student name. We would need to reset the value of 'found = false' inside the while loop:
Steven Parker
230,995 PointsSteven Parker
230,995 PointsThe very first line in my example does reset "found" inside the "while" loop.