Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial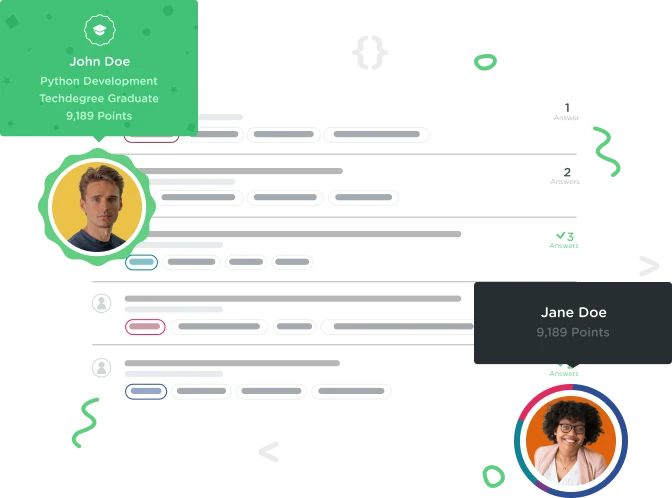
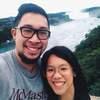
Andrew Wong
Full Stack JavaScript Techdegree Student 15,373 PointsSolution to Extra Challenge at End Of Video
I tweaked Kenan Xin's solution. I found this solution to be very robust. I added found = false; and message = ''; at the end.
var message = '';
var student;
var search;
var found = false;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport ( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: Type a name [Jody] or type "quit" to end');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message += getStudentReport( student );
found = true;
print(message);
}
}
if ( found == false ) {
print('<h2>Sorry, no student named ' + search + ' was found.</h2>');
}
found = false;
message = '';
}
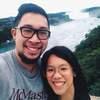
Andrew Wong
Full Stack JavaScript Techdegree Student 15,373 PointsAhh, true. Thanks Andrew!

Unsubscribed User
5,119 PointsAndrew Robinson, in your solution when you print the "Student is not found" line, does it technically print "Student is not found" a number of times even when you search for a name that exists?
Let's say I searched for "Trish" which is the 5th object in the array. Since your if/else statement is inside the for loop wouldn't the HTML print 'Student is not found' 4 times before the loop got to the "Trish" object? Does it just happen so fast that we don't see it? It seems to me that it would loop through that code 4 times before getting to Trish, thus printing "Student is not found". Does that make sense?
Edit: meant to post this as a comment not an answer.
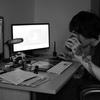
Andrew Robinson
16,372 PointsJeff Jensen:
It would only show one instance of "Student not found." because the print function replaces the innerHTML each time, so technically the print function is being called for every student not found but it's replacing it each time.
On the other hand when we print the array of students found, the listStudent function concats the message for each student, and then the print function prints the entire list of students at once.
Hope that clears things up a bit :)

Unsubscribed User
5,119 PointsThanks for the immediate response! I understand that it's being replaced I just wanted to know if it was in fact printing it once before it was replaced by the found student. So if you had a database of tens of thousands of students you might be able to see the "Student not found" statement printed until the computer finds a match right? Thanks!
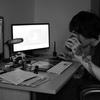
Andrew Robinson
16,372 PointsJeff Jensen
Yes it's definitely not a scalable option, what I'd most likely do differently now is after the for loop check if the found array is empty and if it is print the not found message, at the minute it's calling the print function for every student not found which is a waste of resources, thanks for pointing that out :)

Unsubscribed User
5,119 PointsGotcha! That makes perfect sense, I just wanted to make sure I was reading through the code correctly! I appreciate the replies!
-Jeff
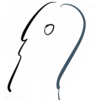
akak
29,445 PointsHere's mine solution without creating additional variable.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message += getStudentReport( student );
}
}
if (message.length > 0){
print(message);
message = '';
} else {
print("There is no such student.");
}
}
I don't know if it's bulletproof, but works for me ;)
Andrew Robinson
16,372 PointsAndrew Robinson
16,372 PointsNice solution, you overlooked one thing though, what if someone doesn't type a name case-sensitive? :)
Here was my solution, slightly different but works just fine.