Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial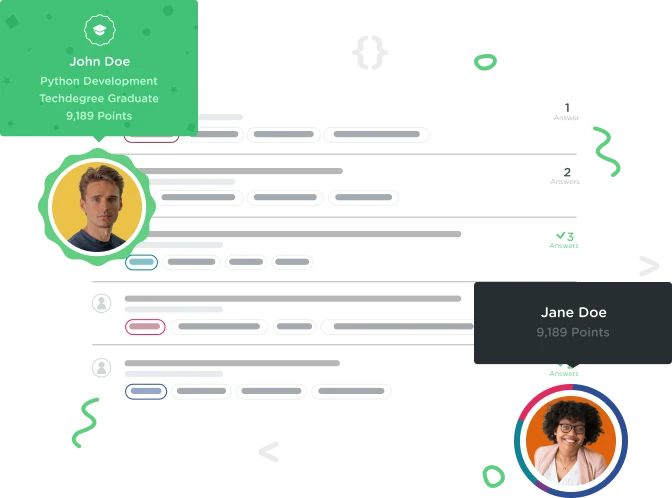

Gareth Moore
7,724 PointsSolution using Arrays to prompt for user input :)
// FUNCTIONS for area and volume calculations
function areaRect(num1, num2) {
const output = num1 * num2;
return(output);
}
function volRect(num1, num2, num3) {
const output = num1 * num2 * num3;
return(output);
}
function areaCir(num1) {
const output = Math.PI * Math.pow(num1, 2);
return(output);
}
function volSphere(num1){
const output = (4/3) * Math.PI * Math.pow(num1, 3);
return(output);
}
// PROMPT user for dimensions of shapes. Store in const array (using function below: inputNum)
const input = [inputNum("width"), inputNum("length"), inputNum("height"), inputNum("radius")];
// FUNCTION for user input and user input evaluation as number
function inputNum(dimension){
let input = prompt(`Please enter the ${dimension} value:`);
while (isNaN(input)) {
input = prompt(`That was not a valid number. Please type a ${dimension} value:`);
}
return(input);
}
// Output the area and volume calculation in html
document.querySelector('body').innerHTML = `
<h1>Here are the results!!</h1>
<p>The area of your rectangle is ${areaRect(input[0], input[1])}</p>
<p>The volume of your rectangle is ${volRect(input[0], input[1], input[2])}</p>
<p>The area of your circle is ${areaCir(input[3])}</p>
<p>The volume of your sphere is ${volSphere(input[3])}</p>`;
//Hope this helped you in some way :)
2 Answers
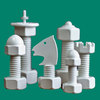
Steven Parker
231,008 PointsVery clever!
But be aware that the return from prompt is always a string. The isNaN test works because the argument is coerced into a number, but some operations could produce unexpected results. For example, if a +
operator were used, it would be interpreted as concatenation instead of addition. To prevent this, you can explicitly convert the input into numbers using parseInt:
let input = parseInt(prompt(`Please enter the ${dimension} value:`));
while (isNaN(input)) {
input = parseInt(prompt(`That was not a valid number. Please type a ${dimension} value:`));
}
Also, it occurred to me that map might provide a clever way to acquire the input:
const input = ["width", "length", "height", "radius"].map(a => inputNum(a));

Gareth Moore
7,724 PointsThanks for your advice Steven :)
Would you mind explaining a little bit more. I am not sure I fully understand you.
My thinking is that whatever string the user inputs via the prompt
function, it will be passed through the isNaN
function. The isNaN
function (as far as I am aware) evaluates a value to see if it is a number variable or not. So if it is false then the user input is a number. If it is true then the user input isn't a number (a string or whatever else it could be). So can the isNaN
function return a value of say +4
as a number variable? Or am I missing something else entirely haha.
Again, thanks for your help!
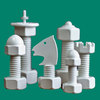
Steven Parker
231,008 PointsSee the comment I added to my answer.
Steven Parker
231,008 PointsSteven Parker
231,008 PointsThe implicit coercion I mentioned before can be confusing and have unexpected results. The isNaN function first tries to convert its argument into a number, and then checks if the result is
NaN
. So a string containing digits will still get afalse
result even though it is a string and not an actual number. Coercion also happens for some math operations, but not if the operator has a different meaning for strings (like+
does).