Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial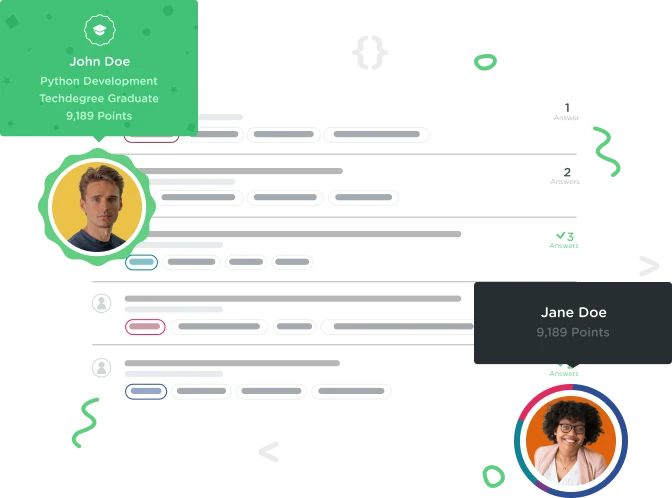
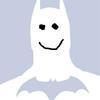
Hugo Porfirio
4,584 PointsSolution with (a little)more output data, feedback appreciated.
// Variables
var answer;
var answers = [[], []];
// Array with questions and answers
var questions = [
["How much is 2+2?", "four"],
["Sky color?", "blue"],
["Worst programming language?", "php"]
];
// Put the content inside the #output div withouth erasing the previous data
function print(message) {
var outputDiv = document.getElementById("output");
outputDiv.innerHTML += message;
}
// Build a proper html list from an array
function buildList(list) {
var listHTML ="<ol>";
for(var i = 0; i < list.length; i += 1) {
listHTML += "<li>" + list[i] + "</li>";
}
listHTML += "</ol>";
return listHTML;
}
//Ask questions=[i][0] and save wrong/correct answers
for (var i = 0 ; i < questions.length ; i += 1) {
answer = prompt(questions[i][0]);
// If correct, store question and answer in the first array inside answers=[[correct answers],[]]
if (answer === questions[i][1]) {
alert("Correct!");
answers[0].push(questions[i][0] + " R: " + answer);
// If wrong, store question, wrong answer and correct answer in the second array inside answers=[[],[wrong answers]]
} else {
alert("Wrong!");
answers[1].push(questions[i][0] + " Your answer: " + answer + " Correct answer: " + questions[i][1]);
}
}
//Print the lists with correct and wrong answers
print("<h2>You got " + answers[0].length + " correct answers:</h2>" + buildList( answers[0] ) );
print("<h2>And " + answers[1].length + " wrong answers:</h2>" + buildList( answers[1] ) );
Well, it works... but I have a feeling that many things are not properly done/the best way. Thanks in advance!