Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial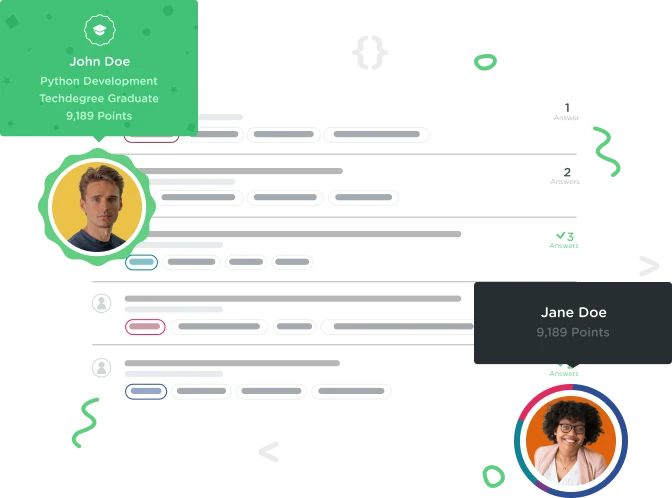
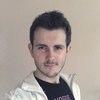
Alex Devero
24,526 PointsSolution with modular design
Here is a solution using modular design pattern:
(function() {
var recordPrinter = {
// Init method for controlling the printer methods
init: function() {
var answer = prompt("Use 'print' to print all records, or 'search' to find specific student.").toLowerCase();
if (answer === "print") {
this.printRecords(students);
} else if (answer === "search") {
this.searchRecords(students);
}
},
// Method for printing records
printRecords: function(array) {
var container = document.getElementById("output");
for (var i=0, j=array.length; i<j; i++) {
for (var prop in array[i]) {
container.innerHTML += "<strong>" + prop + ":</strong> " + array[i][prop];
container.innerHTML += "<br>";
}
container.innerHTML += "<br>";
}
return container;
},
// Method for searching records
searchRecords: function(array) {
var name = prompt("What name do you want to search?");
var container = document.getElementById("output");
for (var i=0, j=array.length; i<j; i++) {
console.log(array[i].name);
if (array[i].name === name) {
for (var prop in array[i]) {
container.innerHTML += "<strong>" + prop + ":</strong> " + array[i][prop];
container.innerHTML += "<br>";
}
}
}
return container;
}
};
// Automatic initialization of recordPrinter obejct's init method
recordPrinter.init();
})();