Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial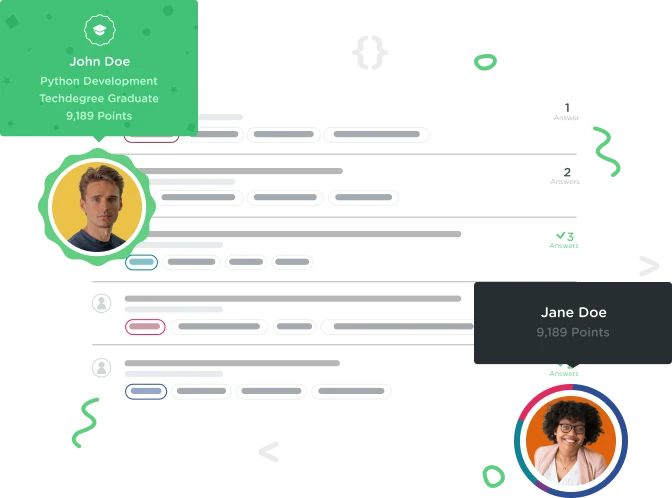
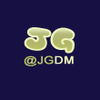
Jonathan Grieve
Treehouse Moderator 91,253 Points[SOLVED] Exception: Cannot convert string type to JSON object
I feel like I'm almost there but I've got a runtime error that i just can't seem to shake off. It seems to be having a problem looking for a particular string, maybe the humidity part of the query?
package uk.co.jonniegrieve.stormy;
import android.app.Activity;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.net.Uri;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.Toast;
import com.google.android.gms.appindexing.Action;
import com.google.android.gms.appindexing.AppIndex;
import com.google.android.gms.appindexing.Thing;
import com.google.android.gms.common.api.GoogleApiClient;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class MainActivity extends Activity {
private static CurrentWeather mCurrentWeather;
public static final String TAG = MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//set up string to query API
String apiKey = "c967557031449620255ff3c64b443a26";
double latitude = 37.8267;
double longitude = -122.4233;
String forecastURL = "https://api.darksky.net/forecast/" + apiKey + "/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
//make a new OkHttpClient object
OkHttpClient client = new OkHttpClient();
//new request object - method chaining
Request request = new Request.Builder()
.url(forecastURL)
.build();
//make a request from a Call class
Call call = client.newCall(request);
//make and asynchronous call with the enqueue method
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
if (response.isSuccessful()) {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
mCurrentWeather = getCurrentDetails(jsonData);
} else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught", e);
} catch (JSONException e) {
Log.e(TAG, "Exception Caught", e);
}
}
});
} else {
Toast.makeText(this, getString(R.string.network_unavailable_message),
Toast.LENGTH_LONG).show();
//code challenge: make into Dialog fragment
}
//log to console
Log.d(TAG, "Main UI code is running!");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast= new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "FROM JSON: " + timezone);
JSONObject currently = new JSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
//return null
//return new CurrentWeather();
Log.d(TAG, currentWeather.getFormattedTime());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager= (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
E/MainActivity: Exception Caught
org.json.JSONException: Value currently of type java.lang.String cannot be converted to JSONObject
at org.json.JSON.typeMismatch(JSON.java:111)
at org.json.JSONObject.<init>(JSONObject.java:160)
at org.json.JSONObject.<init>(JSONObject.java:173)
at uk.co.jonniegrieve.stormy.MainActivity$override.getCurrentDetails(MainActivity.java:105)
at uk.co.jonniegrieve.stormy.MainActivity$override.static$access$100(MainActivity.java:29)
at uk.co.jonniegrieve.stormy.MainActivity$override.access$dispatch(MainActivity.java)
at uk.co.jonniegrieve.stormy.MainActivity.access$100(MainActivity.java:0)
at uk.co.jonniegrieve.stormy.MainActivity$1.onResponse(MainActivity.java:75)
at okhttp3.RealCall$AsyncCall.execute(RealCall.java:135)
at okhttp3.internal.NamedRunnable.run(NamedRunnable.java:32)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1113)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:588)
at java.lang.Thread.run(Thread.java:818)
E/Surface: getSlotFromBufferLocked: unknown buffer: 0xa1f4c0e0
2 Answers

Kudakwashe Blessing Muzividzi
3,031 PointsThis was helpful thanks.

Neji Tee
729 PointsThanks. I ran into the same problem
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsSolved.
It turns out the runtime error stretched back to the previous video.
I was doing
JSONObject currently = new JSONObject("currently");
instead of
JSONObject currently = forecast.getJSONObject("currently");
So my guess is Android thought I was trying to setup an entirely new JSON object instead of trying to retrieve information from an existing one! :) Now the console displays the time perfectly!