Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial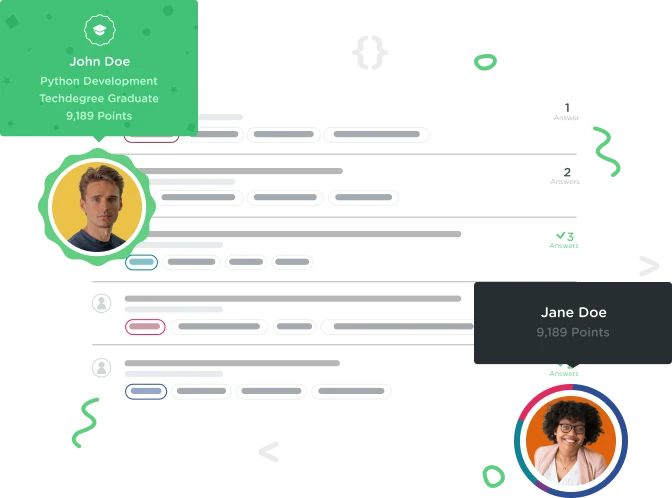
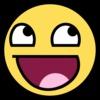
Marcus Schumacher
16,616 Points[SOLVED] Slight Problem With Local Storage Challenge
I am so close!
function getStoredListItems() {
var storedItems = localStorage.getItem('storedItems');
if(storedItems) {
return JSON.parse(storedItems);
}
else {
return [];
}
}
function addStoredItem(str) {
var storedItems = getStoredListItems();
storedItems.push(str);
localStorage.setItem('storedItems', JSON.stringify(storedItems));
}
Those functions seem to work. I successfully add each new List Item text value to storedItems in localStorage. This is exactly what storedItems under localStorage looks like: storedItems: "["Name","AnotherName","YetAnotherName"]"
Here is where the problem is
getStoredListItems().forEach(function(text) {
createLi(text);
});
Nothing happens! No ListItems are created from the text I am given! Please help! For reference, here is the whole dang createLi function:
// This whole function creates a list item with all the required buttons and elements
function createLi(text){
function createElement(elementName, property, value) {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
function appendToLi(elementName, property, value) {
const element = createElement(elementName, property, value);
li.appendChild(element);
return element;
}
const li = document.createElement('li');
appendToLi('span', 'textContent', text);
appendToLi('label', 'textContent', 'Confirm')
.appendChild(createElement('input', 'type', 'checkbox'));
appendToLi('textarea', 'textContent', 'Notes'); // I need to make the display value of this text area block inline
appendToLi('button', 'textContent', 'edit');
appendToLi('button', 'textContent', 'remove');
return li;
}
Are the items I am getting back from getStoredListItems not strings?
1 Answer
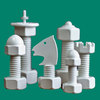
Steven Parker
241,955 PointsYou say "No ListItems are created from the text I am given!", but maybe they are.
The "createLi" function appears to create new list item elements and return them, but when you call it from within the "forEach", nothing is done with the return value. In particular, it's not being added to the DOM. So essentially the created element just exists in "limbo".
I would expect that you'd want to append them to an existing list (ul or ol).
Marcus Schumacher
16,616 PointsMarcus Schumacher
16,616 PointsThank you so much! I can't believe I didn't see that :D
Steven Parker
241,955 PointsSteven Parker
241,955 PointsGlad I could help, and happy coding!