Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial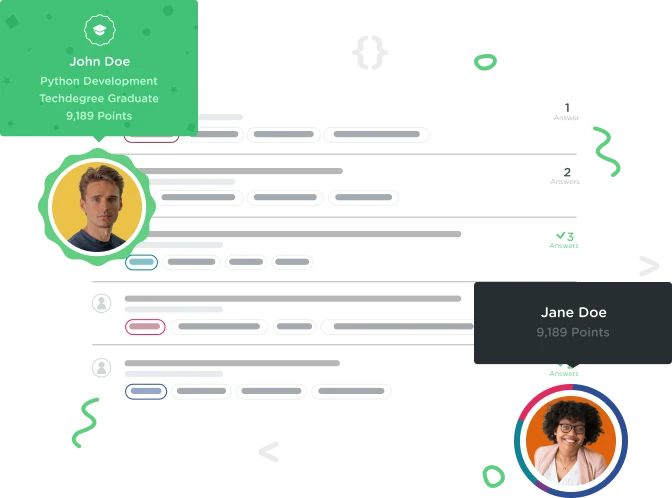
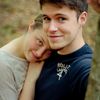
Benjamin Bledsoe
10,900 PointsSpecifics of a Function Question
Okay, at the end of the video, Dave mentions that you could create a function that takes an array as a parameter to average test scores. I decided to do this, and this is what I came up with:
var testScore = [ 97, 55, 87];
function averager( item ){
var tally=0;
var divideBy=0;
for(var i=0; i < item.length; i += 1){
tally+=item[i];
divideBy +=1;
}
var answer = (tally/=divideBy);
Math.floor(answer);
return answer;
}
document.write(averager(testScore));
I have two main questions with this:
1) Why do I need to write '/=' to divide within the answer variable? I tried just using '/' and it didn't work, and I am very curious as to why.
2) Why is Math.floor(answer); not having effect of the final value of the answer variable?
I would greatly appreciate any explanations, and thank you in advance!
2 Answers

Joshua Edwards
52,175 Points1) I got it to work just using '/' so I'm not sure why it did not work for you 2)You need to run Math.floor when you return answer or assign Math.floor(answer) to another variable that you then return. Math.floor doesn't change what is actually stored in the variable.
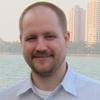
Ryan Field
Courses Plus Student 21,242 PointsI also got it to work by using simply the division operator, but I'm not entirely sure why you're getting a float returned from your function. In any case, I tried the following code in my console, and it seemed to work fine:
var testScore = [ 97, 55, 87];
function averager( item ){
var tally=0;
var divideBy=0;
for(var i=0; i < item.length; i += 1){
tally+=item[i];
divideBy +=1;
}
var answer = (tally/divideBy);
return Math.floor(answer);
}
document.write(averager(testScore));
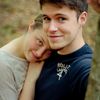
Benjamin Bledsoe
10,900 PointsThanks man, I realized that I didn't use the floor method with return so it did not get stored in the variable
Benjamin Bledsoe
10,900 PointsBenjamin Bledsoe
10,900 PointsThank you, I guess I wasn't fully grasping the scope of storing data in variables with respect to this incident! Thanks for clearing that up!