Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial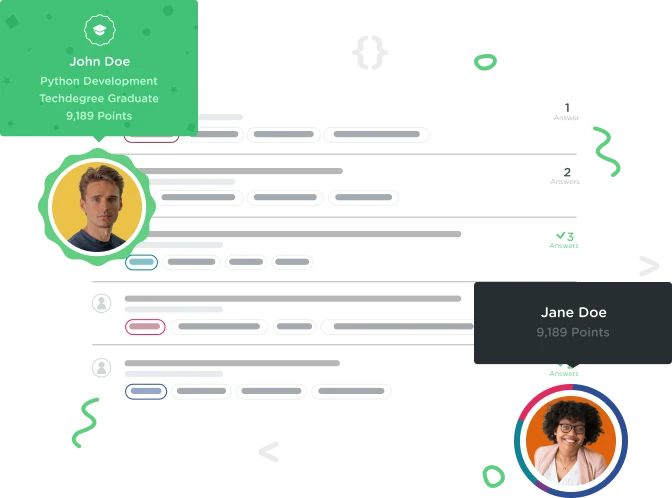
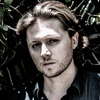
Richard Nash
24,862 PointsStatements, Declarations and Expressions. Which is which?
This is something that is confusing me a little bit...
The following example creates a function:
function myFunc() {
//stuff
}
As well as this one:
var myFunc = function() {
//stuff
}
The second example is called a statement, according to the video. So what is the first example called? I've heard terms like Declaration and Expression thrown around.
Is the first example a declaration or an expression, or something else?
Do those two terms mean the same thing, or different things?
I've also heard the term hoisting thrown around a bit, and this fella named Douglas Crockford, who I've only recently discovered, seems to recommend using the second example above for functions because the first example exhibits problems with hoisting, which i do not really understand at this point.
This is all rather murky to me, and it would be nice if somebody could help shed some light on these subtle, but seemingly important distinctions.
On the surface, the first example seems more clear and logical, but i would like to establish a best practice from the beginning of my learning so that I'm good to go into the future.
Thank you for your time XD
Richard Nash
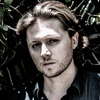
Richard Nash
24,862 Pointshmmmm... found another article that uses the terms in the opposite combination:
http://www.unicodegirl.com/function-statement-versus-function-expression.html
This article explains that function "statements" are when you write the keyword function and then the name of the function.
But in the video in the treehouse course and in the other article I posted in the previous comment the term "statement" is used to name the second example where a variable is declared and then assigned an anonymous function.
As somebody learning the language this is frustrating and inconsistent. So what is the answer?
statement = declaration?
statement = expression?
declaration = expression?
or statement != expression != declaration?
I don't know what to think here :-(
4 Answers
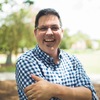
Dave McFarland
Treehouse TeacherHi Richard
If a line begins with function
it is a declaration; otherwise it is an expression.
Declaration:
function sayHi() {
alert('Hi!');
}
Expression:
var sayHi = function() {
alert('Hi!');
};
Notice that there's a semicolon at the end of a function expression -- because this is really an assignment to a variable this code is a statement and should have a semicolon at the end.
The main difference between the two is that a function declaration can be placed ANYWHERE in a script including at the bottom of the script. The browser's JavaScript interpreter reads the script before running anything and commits the function to memory. Because of this it can be called anywhere in the script -- even BEFORE the declaration actually appears in the script. For example, this works:
sayHi();
function sayHi() {
alert('Hi');
};
A function expression, on the other hand, is only created during runtime -- in other words you can't call the function before until the JavaScript interpreter evaluates (or runs) the expression. For example this WON'T work"
sayHi();
var sayHi = function() {
alert('Hi');
};
To avoid all this confusion, it's often a good idea to declare your functions at the beginning of a script when possible.
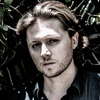
Richard Nash
24,862 PointsSo whether it's a declaration or an expression it's best to declare functions up front to avoid any issue when the browser reads your code then?
Still a little hazy on what a "statement" is then, but this is helping a bunch XD

Lonnie Wibberding
18,318 PointsHi Richard,
Good questions.
So the first example is a declaration because you are "declaring" here is a function!
The second one, you are actually not naming the function, but putting it in a variable with a name. This, I believe does have some implications you have mentioned with hoisting.
Hoisting is the way JavaScript processes variable. Say you have a function as follows
var myFunc = function() {
//stuff
//more stuff
// even more stuff
var aVariable = 100;
}
JavaScript will process this as if the variable was declared at the top of the function. This is hoisting. Taking the variable and declaring them at the top. Simple process, scary name. The code will be processed like this:
var myFunc = function() {
var aVariable;
//stuff
//more stuff
// even more stuff
aVariable = 100;
}
Anyway, hope this helps . . .
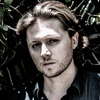
Richard Nash
24,862 PointsThank you Lonnie Wibberding, this is helping me a bunch XD
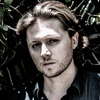
Richard Nash
24,862 PointsThe course was great, by the way. It helped clear up a few hazy concepts in my head. Looking forward to the next course on loops, arrays and objects.
I'll stop bugging you now, go have candy! XD
And thanks again!
R
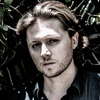
Richard Nash
24,862 PointsI've copied this verbatim from the following article:
http://www.unicodegirl.com/function-statement-versus-function-expression.html
Some of the assertions in the article are a little confusing, so I have emphasized my own comments and question throughout the article.
Begin Article...
Function Smackdown: Function Statement vs. Function Expression
I’ve recently been reading ‘JavaScript: The Good Parts,’ by Douglas Crockford. This concise book defines a subset of core JavaScript that’s robust and powerful: The Good Parts. Appendix B identifies The Bad Parts, and sets our competitors - the function statement and the function expression - apart.
ROUND ONE - LOOKS (CAN BE DECEIVING)
Function Statement (does this person mean declaration?)
Our competitors may look alike, but the function statement has two distinguishing characteristics: the declarative keyword function always comes first, and you must name it. Here’s what it looks like:
//what we see:
function funcName(){
//stuff
}
Pull back the curtain (what curtain?) , though, and the function statement expands into a variable with a function value:
// Under the hood
var funcName = function funcName(){
//stuff
}
Function Expression
Absent the mark of the function statement, you have the function expression. The function expression is optionally named (but normally anonymous), e.g.:
// Stores ref. to anonymous function in a variable
var funcRef = function(){
//stuff
};
// Stores ref. to named function in a variable
var funcRef = function funcName(){
//stuff
};
WINNER: Function Expression. Crockford’s manual argues the case for clear code. The function expression is clearly recognisable as what it really is (a variable with a function value). is this what the browser does to a function? and do all browsers behave similarly?
ROUND TWO - (UNPREDICTABLE) BEHAVIOUR
Function Expression
Variables are subject to a thing called hoisting. Irrespective of their apparent place in the flow of things, their var part is removed and hauled to the top of a containing (whether function or global) scope, and initalised with undefined: (is this part of the 'under the hood' part?)
// What we see
var funcRef = function(){
//stuff
};
// Under the hood
var funcRef = undefined;
funcRef = function(){
//stuff
};
Function Statement (again, declaration?)
The function statement - while just shorthand for a var statement with a function value - is treated differently: the whole lot is hoisted. For this reason, you can call a function statement before you have declared it in your code:
console.log(statement()); // I am a function statement.
console.log(expression()); // TypeError...
function statement(){
return "I am a function statement.";
}
var expression = function(){
return "I am a function expression.";
}
WINNER: Function Expression. Hoisting is already a behind-the-scenes behaviour that can cause head scratching. The particular behaviour of the function statement can lead to more furious head scratching.
ROUND THREE - SUPER POWERS
Function Statement (you know.)
The function statement is widely used and performs just fine, but it has an obvious limitation: You can’t immediately invoke it.
Function Expression
The function expression is more flexible, e.g.:
// Executed immediately
(function() {
alert("I am not a function statement.");
}());
Parentheses are required to nudge function out of statement position (can’t immediately invoke), and into expression position (can immediately invoke). Crockford recommends grouping the entire invocation inside parentheses for clarity - what’s important here is the product of the invoked function - otherwise, Crockford argues, “you’ve got these things hanging outside of it looking like dog balls.”
WINNER: Function Expression.
FINAL SCORE: Function Expression Wins 3 - 0
CONCLUSION - I HEART A GOOD MANUAL
It turns out the function statement came first; the function expression was added to JavaScript later. The result is two very similar ways of defining JavaScript functions. Logically, the addition was intended to improve the language, and, in this case, it would seem this end was achieved.
... End Article.
So, this is all very interesting and confusing and making my head run around in circles about all this stuff. Just curious to get everybody's thoughts on these assertions and try to get a more balanced perspective.
Again, thank you! XD
Richard Nash
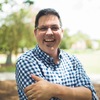
Dave McFarland
Treehouse TeacherHi Richard
Declaration (or statement):
function sayHi() {
alert('Hi!');
}
Expression:
var sayHi = function() {
alert('Hi!');
};
Both are ways of creating functions, and both are commonly used. Some people like expressions -- but that's really just a personal preference. The important thing is the slight difference in behavior as described in the posts above.
You're right -- I made a mistake in one of my videos and mistakenly called a function expression a function statement! We'll get that fixed. Thanks for pointing it out.
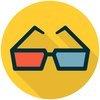
kevinardo
Treehouse Project ReviewerHi guys, thx for some great posts.
A question for Dave the Great! :) Why is there a param after the variable name in your Expression example above?
Looking forward to alot more JavasCript courses from Treehouse.
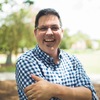
Dave McFarland
Treehouse TeacherWhy? Because I messed up :)
Thanks for pointing that out. I removed those parentheses after the var name in the expression examples from above.
Perhaps "Dave the not always great" is a better nickname for me!
Richard Nash
24,862 PointsRichard Nash
24,862 PointsJust found this site:
http://bonsaiden.github.io/JavaScript-Garden/
It attempts to explain some of the quirky elements of javascript and talks about this question. They use the term "expression" in place of the term "statement" to describe my second example where a variable is declared and then assigned an anonymous function.
So I guess function declarations are when you write the keyword "function" and follow it with the name of the function plus the parens for arguments and then the curly braces.
When you use the syntax of my second example then it is called either a "statement" or an "expression."
I've also read about literal notation a bit, but it is still a little murky. I'm wondering if function declarations could be called "function literal notation".
Do you guys have any advice on these questions Dave McFarland, Andrew Chalkley and/or Jim Hoskins?
Again, thanks for all your great work and energy :-)
R