Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial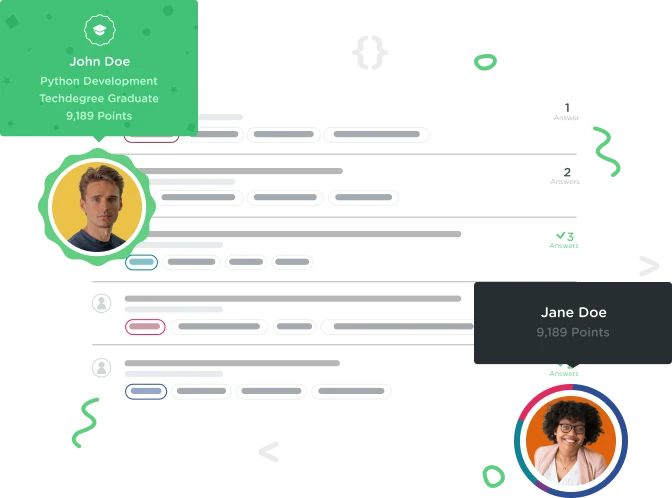

Shefeek N
1,303 Pointsstats
Why doesn't this work
def stats(dict):
result = list()
for key in dict:
result.append([key, len(dict[key])])
return result
def stats(teacher):
result = list()
for key in teacher:
result.append([key, len(teacher[key])])
return result
1 Answer
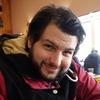
Eric M
11,546 PointsHi Shefeek,
For your first code snippet dict
is a keyword in Python, so it's not great to use as a name in our programs.
A dictionary is a key value store, so you need to operate on both in a single stats function to return details about both the key and the value. Luckily Python offers an easy way to do this:
def stats(our_dictionary):
for key, val in our_dictionary.items():
Unlike a list (where we could just write for item in our_list
) we need to specify that we want the dictionary's items. We want to look at each key and value as a pair.
In this way inside our loop we can iterate over the key value store using our two identifiers (in this example key
and val
).
This this challenge our keys are strings and our values are lists, so an entry will look something like:
"Bobby" : ["first list item", "second list item"]
For the final step of this challenge it is necessary to create a new list, that within it contains several lists, each of those lists should have the first list item being the key from our dictionary, and the second list item should be an integer containing the number of items in the list in the dictionary. <- Not a great explanation! Sorry!
It's probably easier to see in example, our Bobby key val becomes: ["Bobby", 2]
then we append that to a new list, once we've done the append for all of our iterations we can return the new list.
It looks something like this:
def stats(foo):
result_list = list()
for key, val in foo.items():
new_list_item = [key, len(val)]
result_list.append(new_list_item)
return result_list
Shefeek N
1,303 PointsShefeek N
1,303 PointsThanks emck for your time.