Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial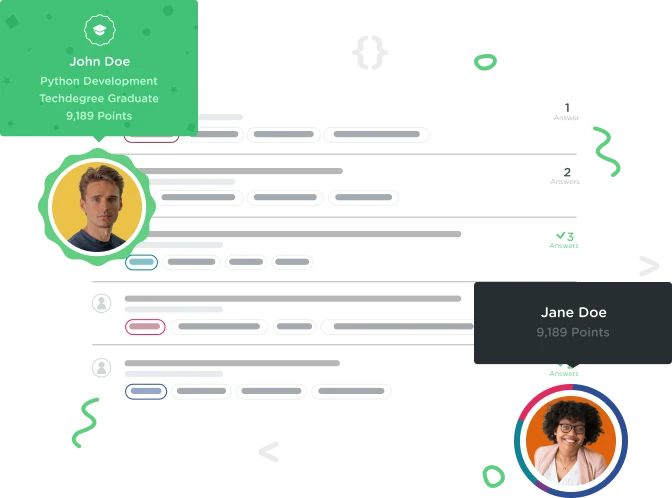

Michael Helgesen
1,140 PointsStep by step describing the script
Hi folks! I am trying to wrap my head around this code. Could you please give me some insight and comment if I have the correct understanding?
// 1. Create the array playList with items.
var playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
// 2. Create a message? Where does this come in? What does this do? A global scope?
function print(message) {
document.write(message);
}
// 3. We create a function, but why do we put 'list' here? Is it a name? But isnt 'printList' the name?
function printList ( list ) {
// 4. We create a var that creates our ordered list.
var listHTML = '<ol>';
// 5. We create a for loop that changes the content(or parameter? What is the right term?) of 'listHTML' so that it now creates a list item to the ordered list. But what does the '[i]' mean? And how can we use 'list.lenght' here? How come 'list' is populatet? Shouldnt it be playList.lenght?
for ( var i = 0; i < list.length; i += 1) {
listHTML +='<li>' + list[i] + '</li>';
}
// 6. We again change the listHTML var to close the ordered list
listHTML += '</ol>';
// 7. We print the function 'printList' to the page?.
print(listHTML);
}
// 8. Or is this the print?
printList(playList);
Really appreciate if you could help a noob from Norway trying to figure out this loops and arrays :)

Michael Helgesen
1,140 PointsThanks Steven. I was not sure how to do it. Great to know. Makes is pretty much more readable!
3 Answers

andren
28,558 PointsCreate the array playList with items.
var playList = [ 'I Did It My Way', 'Respect', 'Imagine', 'Born to Run', 'Louie Louie', 'Maybellene' ];
Your understanding is correct.
Create a message? Where does this come in? What does this do? A global scope?
function print(message) { document.write(message); }
That line of code creates a function called print
. message
is a parameter for the function, meaning that you can pass data into it when the function is called. Code within a function is not run until the function is called in the code. The message
variable within the function will be set equal to whatever data is passed into the function when it is called.
The function itself simply calls document.write
with the value that was passed into the function when it is called.
We create a function, but why do we put 'list' here? Is it a name? But isnt 'printList' the name?
function printList ( list ) {
printList
is indeed the name of the function. list
is the name of the parameter. Parameters are basically variables that are populated when the function is called. So list
will be assigned whatever value is passed to the printList
function when it is called. Values that are passed to a function is called an argument.
We create a var that creates our ordered list.
var listHTML = '<ol>';
It is a var that will end up containing the ordered list.
We create a for loop that changes the content(or parameter? What is the right term?) of 'listHTML' so that it now creates a list item to the ordered list. But what does the '[i]' mean? And how can we use 'list.lenght' here? How come 'list' is populatet? Shouldnt it be playList.lenght?
for ( var i = 0; i < list.length; i += 1) { listHTML +='<li>' + list[i] + '</li>'; }
Content is the more appropriate right term. You can pull out data from a list by referencing the index of the data you want to pull out within square brackets. And indexed start at 0. So list[0]
for example would pull out the first item of the list. list[1]
would pull out the second item from the list and so on.
i
is a variable that starts at 0 within the loop and is increased by one each time the loop runs. by using that as an index to pull from you end up pulling out the first item from the list the first loop, the second item in the second loop and so on. All of the items in the list ends up being pulled out before the loop ends.
list
is already populated due to it being a parameter as I explained above, it will contain whatever argument is passed in to the function when it is called. Passing data into a function through a parameter makes it more flexible than having the function refer to data outside of it.
We again change the listHTML var to close the ordered list
listHTML += '</ol>';
Correct.
We print the function 'printList' to the page.
print(listHTML); }
Not quite. You call the function print
and pass it the listHTML
variable as an argument. Meaning that the message
parameter within the print
function will be set to the contents of listHTML
when the code within it runs.
You didn't ask about the final line but I'll include an explanation for completeness:
printList(playList);
This line calls the printList
function with playList
as an argument. So list
within the printList
function will contain the content of playList
.
This explanation was written somewhat quickly and I tried to be somewhat succinct since there were a lot of questions to go through. If you want more details on any of these points or found part of my explanation unclear then feel free to reply with follow up questions. I'll gladly answer any question that I can.

andren
28,558 PointsThank you. Since we are both pretty active we are bound to collide every now and then .
Your answers tend to be quite a bit more succinct than my answers, and we tend to explain things differently so I don't think it's a bad thing to have answers from both of us. We each offer useful advice in our own way.
And congrats to you for passing the 70K mark .
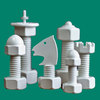
Steven Parker
230,995 PointsNo, not a bad thing, but your answers are reliably accurate and complete so I wouldn't intentionally answer the same question.

Michael Helgesen
1,140 PointsI dont mind getting answers from two brilliant minds at once! :D Its fantastic

Michael Helgesen
1,140 PointsAha... its so great to get feedback and help. Thanks a lot Steven Parker and andren . This makes it more clear. So, if I understand correctly:
function print(message) {document.write(message);} This creates a shortcut of sorts so that we can write 'print()' and actually get a document.write. The parameter name is just a placeholder for whatever you want to write to the document.
function printList (list) And here 'list' is also a placeholder (the names of these placeholders makes more sense now, as I see that they indicate what they are supposed to do or contain)
The [i] is for retrieving an index in the array., and since its in a for loop, it will go from 0 to 5 in this case.
print(listHTML) This is just short for document.write(listHTML) and creates the ordered list. But since the function isnt called yet, nothing is visible.
printList(playList) Now were talking. This is what creates it all. We call the function, and we put 'playList' as a parameter. That means: It takes all object in the array, puts it into the printList function. The function then is asked to go through everything in that array, and output all those objects as list items. When thats done, the function in the end creates an HTML ol list and write it to the webpage.
Absolutely fantastic when it all comes together (if I understood it that is).
Just one more thing. When we create the var listHTML
we first define that it contains an <ol>. Then we overwrite it to contain <li>s and in the end we tell it to contain </ol>. So we never actually overwrite, we just change the value?
Thanks so much guys. Really really helpful. I learned so much right now!
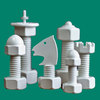
Steven Parker
230,995 PointsIt seems like you've mostly got it.
But the ordered list tag in listHTML
is not overwritten. The "+=" operator is a "concatenating assignment" which puts the new string value after what is already stored there. So each time through the loop it gets larger.
Otherwise, good job!

Michael Helgesen
1,140 PointsEven tho you explained how to format the code Steven Parker I still got it wrong lol. Oh well. Ill try again next time. Sorry

Michael Helgesen
1,140 PointsOf course Steven Parker . I totally overlooked that. It just adds of course! Thanks again!
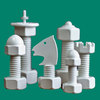
Steven Parker
230,995 PointsI believe I was able to pick out your questions:
2.
Create a message? Where does this come in? What does this do? A global scope?
The function is named "message" but all it does is put a string on the web page. The function is available in the global scope.
3.
We create a function, but why do we put 'list' here? Is it a name? But isnt 'printList' the name?
The name "list" here is the function parameter. It is a placeholder for the real term (such as "printList") that will be used when the function is called later.
5.
We create a for loop that changes the content(or parameter? What is the right term?) of 'listHTML' so that it now creates a list item to the ordered list. But what does the '[i]' mean? And how can we use 'list.lenght' here? How come 'list' is populatet? Shouldnt it be playList.lenght?
The loop changes the content of listHTML
, by adding list items to the unordered list. The variable "i" is the index that the loop uses to get each item, one at a time. Putting a value in brackets("[]
") after an array name is called subscripting or indexing and is how you get one single item from the array. The term "list" is still the placeholder for the real list that will be used when the function is called.
Steven Parker
230,995 PointsSteven Parker
230,995 PointsWhen posting code, be sure to format it using the instructions from the Markdown Cheatsheet found below the "Add an Answer" area