Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial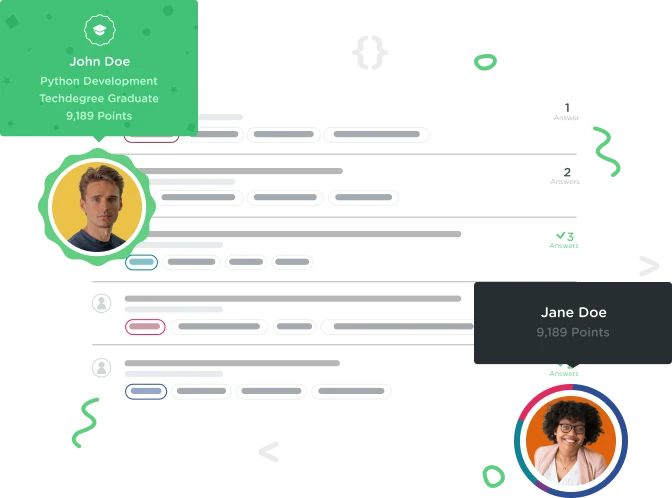
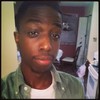
abou93
14,319 Pointsstill very confused in challenge 1 of 4 about dictionary of teachers and most classes
I'm asked to create a function called most_classes and return the teacher with the most classes. now i passed 1 of 4 luckily, but I'm confused as to which teacher or item in teacher_dict is assigned to who and how the variable busy_teacher knows which teacher is teacher1. I thought teacher1 would be assigned to the first item in the list. if that is the case how does busy_teacher automatically become the item with the most classes.
here is my code:
The dictionary will be something like:
{'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
Often, it's a good idea to hold onto a max_count variable.
Update it when you find a teacher with more classes than
the current count. Better hold onto the teacher name somewher
too!
Your code goes below here.
teacher_dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'], 'Kenneth Love': ['python Basics', 'Python Collections']}
def most_classes(teacher_dict): max_count = 0 busy_teacher = '' for teacher1,teacher2 in teacher_dict.items(): if len(teacher2) > max_count: max_count = len(teacher2) busy_teacher = teacher1 return busy_teacher
2 Answers
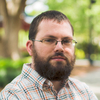
Kenneth Love
Treehouse Guest TeacherSo, when we start out, our max_count
is 0 and our busy_teacher
isn't anyone, it's an empty string. Cool.
Then we start going through the .items()
tuples in our dictionary. The first time through, since that teacher has more classes than 0, the count gets updated to however many classes they have and the name gets set to their name.
Now, every iteration after that, we have an actual comparison. Does this new teacher have more classes than the old one? No, move on. Oh, they do? Then let's change both of these variables to match the newest teacher.
Lather, rinse, repeat until the dictionary is done.
teacher1
and teacher2
in your example code change for every step of the loop. That's how we can do this comparison.
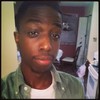
abou93
14,319 PointsAh thank you! so this means that teacher1 and teacher2 are not linked to a specific item in the dictionary? I guess i was confused because it appears as if the compiler goes through, and simply chooses a random item and checks to see if both items are greater than zero, if i changed the name the name of teacher 1 instead to "verybusyteacher" and teacher2 to "notsobusyteacher" i think i see more cleary whats going on. it simply takes one item to see if its greater than zero, if it is, that not so busy teacher and the length of its values becomes the max count then busy teacher must be the other teacher and it does this until its went through each item in the dictionary.
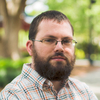
Kenneth Love
Treehouse Guest Teacherteacher1
and teacher2
are linked to specific items in the dictionary (or, rather, a teacher and their classes) but only for however long the code in the for
loop takes to evaluate. Then they're both given two new values.
It's like doing:
for num in [1, 2, 3, 4, 5]:
print(num)
That's going to print each of those numbers. At each step of the for
loop, each time the print()
is called, num
has a new value.
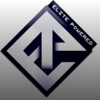
Abenezer Mamo
5,821 PointsThis is my solution to this problem. It's quite simple to understand and it's efficient.
def most_classes(my_dict):
teachers_list = {}
for teacher in my_dict:
teachers_list[teacher] = len(my_dict[teacher])
return max(teachers_list, key=teachers_list.get)
abou93
14,319 Pointsabou93
14,319 Pointswow that code was badly entered, lemme try it again heres my code: