Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial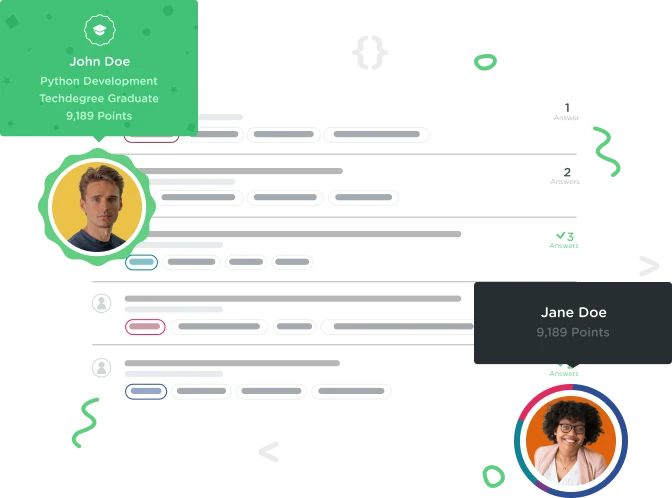

Carlos Quevedo
5,911 Points[Stormy] Day List Layout UI Not Updating
For some reason. Tapping on the 7 Day button brings up a list that only loads 7 "Sundays". Also, the List item padding is wrong so each item looks super small on the list. Any ideas as to what's causing this?
I'm pasting my Day activity code here but let me know if you need some more context. Thanks!
package com.vandalayindustries.stormy.weather;
import android.os.Parcel;
import android.os.Parcelable;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
//model class for the weather for the whole day
public class Day implements Parcelable {
private long mTime;
private double mTemperatureMax;
private String mSummary;
private String mIcon;
private String mTimeZone;
public long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public int getTemperatureMax() {
return (int) Math.round(mTemperatureMax);
}
public void setTemperatureMax(double temperatureMax) {
mTemperatureMax = temperatureMax;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
public String getIcon() {
return mIcon;
}
public void setIcon(String icon) {
mIcon = icon;
}
public String getTimeZone() {
return mTimeZone;
}
public void setTimeZone(String timeZone) {
mTimeZone = timeZone;
}
public int getIconId() {
return Forecast.getIconId(mIcon);
}
public String getDayOfTheWeek() {
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
formatter.setTimeZone(TimeZone.getTimeZone(mTimeZone));
Date dateTime = new Date(mTime * 1000);
return formatter.format(dateTime);
}
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeLong(mTime);
dest.writeString(mSummary);
dest.writeDouble(mTemperatureMax);
dest.writeString(mIcon);
dest.writeString(mTimeZone);
}
private Day(Parcel in) {
mTime = in.readLong();
mSummary = in.readString();
mTemperatureMax = in.readLong();
mIcon = in.readString();
mTimeZone = in.readString();
}
public Day() {
}
public static final Creator<Day> CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel source) {
return new Day(source);
}
@Override
public Day[] newArray(int size) {
return new Day[size];
}
};
}
2 Answers

Seth Kroger
56,413 PointsFor mTemperatureMax you use writeDouble() to Parcel it but readLong() to pull it out. You should use readDouble() so the types match. If you still have trouble after that show us your DayActivity and DayAdapter code.

Carlos Quevedo
5,911 PointsThanks! I guess I typed all that up too fast while following along with the video. I made that change now the app keeps crashing whenever it's trying to sync with the API (my handy error message keeps popping up). All the monitor is giving me is "finishComposingText on inactive InputConnection"
Either way, here is my DayActivity code:
package com.vandalayindustries.stormy.ui;
import android.app.ListActivity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Parcelable;
import android.support.design.widget.FloatingActionButton;
import android.support.design.widget.Snackbar;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.view.View;
import android.widget.ArrayAdapter;
import com.vandalayindustries.stormy.R;
import com.vandalayindustries.stormy.adapters.DayAdapter;
import com.vandalayindustries.stormy.weather.Day;
import java.util.Arrays;
public class DailyForecastActivity extends ListActivity {
private Day[] mDays;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_daily_forecast);
Intent intent = getIntent();
Parcelable[] parcelables = intent.getParcelableArrayExtra(MainActivity.DAILY_FORECAST);
mDays = Arrays.copyOf(parcelables, parcelables.length, Day[].class);
DayAdapter adapter = new DayAdapter(this, mDays);
setListAdapter(adapter);
}
}
And here is my DayAdapter code:
package com.vandalayindustries.stormy.adapters;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.vandalayindustries.stormy.R;
import com.vandalayindustries.stormy.weather.Day;
public class DayAdapter extends BaseAdapter {
private Context mContext;
private Day[] mDays;
public DayAdapter(Context context, Day[] days) {
mContext = context;
mDays = days;
}
//gets us the count of the items in the Day[]
@Override
public int getCount() {
return mDays.length;
}
//grabs an item at the position specified within the array
@Override
public Object getItem(int position) {
return mDays[position];
}
@Override
public long getItemId(int position) {
return 0; //used to tag items for easy reference
}
//where the mapping occurs
//pulls the latest item from the list
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
//brand new
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
//sets a tag for the view that can be reused below
convertView.setTag(holder);
}
else {
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
if(position == 0) {
holder.dayLabel.setText("Today");
}
holder.dayLabel.setText(day.getDayOfTheWeek());
return convertView;
}
private static class ViewHolder {
ImageView iconImageView; //public by default
TextView temperatureLabel;
TextView dayLabel;
}
}
Would could I be missing?