Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial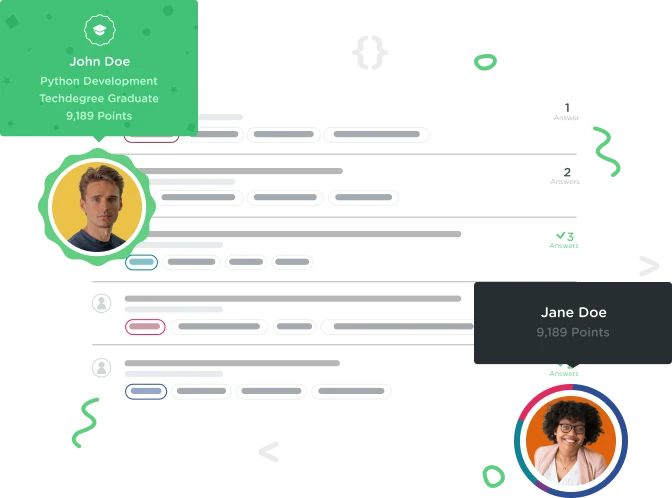

Andrew Ge
2,158 PointsStrange Error Message
The goal of the assignment (if it is not explained somewhere on this page) is to make a method that takes two iterables and returns a single list, with each item in the list a tuple of two elements: the nth element from the first iterable and the corresponding element from the other iterable.
So the final product looks like: [(1st of iterable 1, 1st of iterable 2), (2nd of iterable 1, 2nd of iterable 2), ... ]
The error is telling me that instead of getting the tuples in the order of (10, 'T'), it is receiving (10, 'T').

Andrew Ge
2,158 Pointsdef combo(l1, l2):
tobereturned = []
for n in l1:
for e in l2:
tup = (n, e)
tobereturned.append(tup)
return tobereturned
(strange... I thought I had checked the box that says "include code"; isn't that the default setting? I could have sworn I did not touch it)
[MOD: fixed formatting -cf]
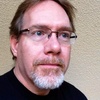
Chris Freeman
Treehouse Moderator 68,460 PointsThe add code button is not working correctly.
2 Answers
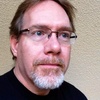
Chris Freeman
Treehouse Moderator 68,460 PointsYour code is creating n**2 tuples instead of n tuples. The first element of l1
is paired with every element of l2
. You want to pull one item from each list and pair them:
def combo(l1, l2):
tobereturned = []
for indx, n in enumerate(l1):
tup = (n, l2[indx])
tobereturned.append(tup)
return tobereturned
# you can also use `zip`
def combo(l1, l2):
return list(zip(l1, l2))
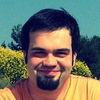
Vladan Grubesic
3,855 PointsI tried few solutions to this problem and I get the same error. "Expecting (10, 'T'), got (10, 'T')."
I googled for the solution and found this code that works:
def combo(iter1, iter2):
combo_list = []
for index, value in enumerate(iter1):
tuple = value, iter2[index]
combo_list.append(tuple)
return combo_list
Original solution was posted by: Pracheeti
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsCan you post your code?