Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial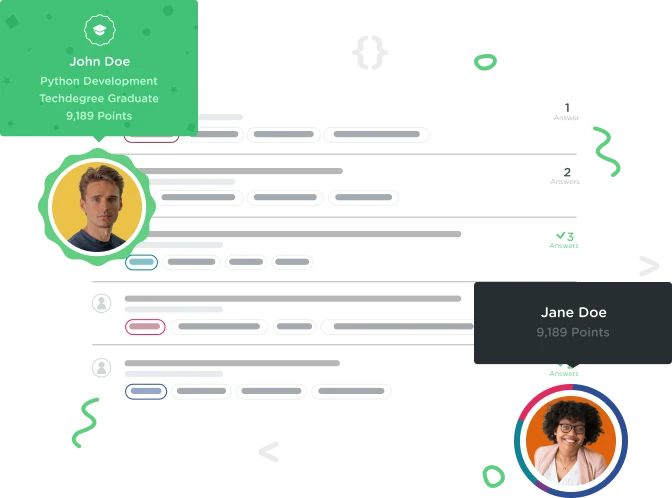

Alexander Bilton
1,982 PointsString_factory
I have no idea how to solve this challenge, can somebody tell me if i'm on the right track?
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory([{"name":"Alexander","food":"Pizza"},{"name":"Andreas","food":"Burger"}]):
return [template.format(name,food),template.format(name,food)]
4 Answers

Elad Ohana
24,456 PointsHi Alexander,
Your new function now has mismatching values that it's passing into the template. Since the template is looking for the keys of "name" and "food" but is getting "name1"/"name2" and "food1"/"food2". I am will give you the framework to my solution with comments to explain my process. Hopefully this helps.
def string_factory(kwargs): # the function accepts a single argument (which should be a list of dictionaries)
# create a temporary empty list for the function
# iterate (loop) through the list of dictionaries, so each variable is the value of each dictionary in the list
# append new strings within the list which include the filled in template with the unpacked dictionary with the **
return () # returns the temporary list as the value
The function should be able to accept a list with any number of different values. The only limitation is that the list should have one or more dictionaries with keys of "name" and "food". Let me know if you need more help.
Elad.

Elad Ohana
24,456 PointsHi Alexander,
The challenge is set to define a function that can take any dictionary and return the completed template in a list. The example in the comments is not meant to be the actual values of the function, but those could be used to test it out. So in essence, you should be able to pass any list of dictionaries with the keys of "name" and "food" to get the desired results. Once you pass the list, you have to iterate through it to get the values from each dictionary into the template string. The current return statement in your function does not have any way to identify which "name" or "food" keys you are referring to (since it does not look at them in any specific order). See if you can figure it out from here. Happy to help if you need more advice.
Elad.

Alexander Bilton
1,982 PointsHi Elad,
Thanks for your reply. What I get from what you're saying is that the return statement needs to be able to differentiate between the keys in that's being passed. Therefore i changed them, but it still does not seem to work... I could use a little more help :-)
''' python
Example:
values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
string_factory(values)
["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory([{'name1':'alexander','food1':'pizza'},{'name2':'andreas','food2':'burger'}]): return [template.format(name1,food1),template.format(name2,food2)]
'''

Alexander Bilton
1,982 PointsA tip for adding code in comments will also be appreciated... Elad Ohana
Alexander Bilton
1,982 PointsAlexander Bilton
1,982 PointsThis helped me the right way - I think I didn't quite understand the **kwargs, which was why I couldn't seem to get it right. Thank you very much