Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial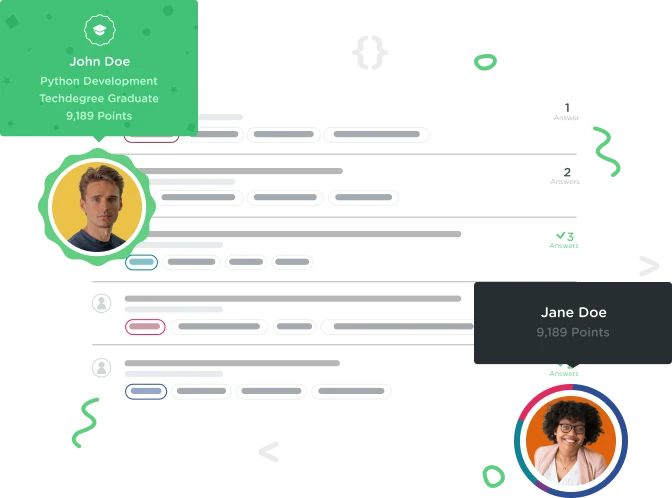

Hwang Ju
2,847 Pointsstring_factory help I am still getting errors and I do not know why..
Let's test unpacking dictionaries in keyword arguments. You've used the string .format() method before to fill in blank placeholders. If you give the placeholder a name, though, like in template below, you fill it in through keyword arguments to .format(), like this: template.format(name="Kenneth", food="tacos") Write a function named string_factory that accepts a list of dictionaries as an argument. Return a new list of strings made by using ** for each dictionary in the list and the template string provided.
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts):
result_list = []
for item in dicts:
result_list.append(string.format(**dicts))
return result_list
9 Answers
William Li
Courses Plus Student 26,868 Pointsbecause item in your for loop is dictionary, dicts is not, it's a list of dictionaries. Therefore, string.format(**dicts)
is wrong both syntactically & semantically, as you can't unpack a list using the ** notation.
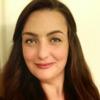
Jennifer Nordell
Treehouse TeacherHi there! You're so close here! So I'm just going to give some hints.
- You're trying to format the string with the list of dictionaries
- Try formatting the string with the current
item
in the list
Hope this helps, but let me know if you're still stuck!
edited for accuracy

Hwang Ju
2,847 PointsThank you it worked!! BUT I'm still having a hard time understanding why that worked. Could you please explain?
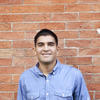
Wilson Usman
35,206 PointsMake sure the indentation is correct, here's what I did:
def string_factory(values):
result_list = []
for item in values:
result_list.append(template.format(**item))
return result_list
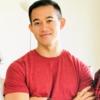
Xayaseth Boudsady
21,951 PointsHere's a simpler way based on the video.
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format(**dict)
Just use the ** (asterisk) to unpack your dictionary into those template.

Hwang Ju
2,847 PointsThank you very much! Happy holidays!
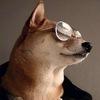
Jerimiah Willhite
12,014 PointsHi! I'm very slowly understanding dictionaries, but not quite getting it. Here's my code:
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}, {"name": "Kenneth", "food":"tacos"}]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(values):
result_list = []
for item in values:
result_list.append(template.format(**item))
return result_list
The error I'm getting is : Bummer! Didn't get all of the expected output from string_factory()
. I'm not sure what's going on that I'm doing wrong. Can anyone help?

Nafeez Quraishi
12,416 PointsHi! you seem to returning within for loop, please try correcting the indentation to return to the function

Tinotenda Muyengwa
3,464 Pointsstring_factory = {"name": "tinomac", "food": "sadza"} """Hi, I'm { } and I love to eat { }!""".format(**string_factory) help guys
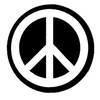
john larson
16,594 PointsTinotenda, did you ever get this one figured out?

Brecht Philips
8,863 Pointshi here is my code challenge with more explainable names template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(list_of_dictionaries):
result_list = []
for dictionary in list_of_dictionaries:
result_list.append(template.format(**dictionary))
return result_list
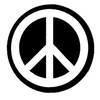
john larson
16,594 PointsNice :D

Kafe Hezam
11,070 PointsShort answer:
def string_factory(iterable): new_list = [template.format(**item) for item in iterable] return new_list